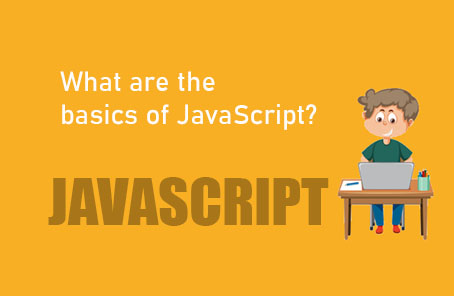
Javascript is a programming language. High-level programming language. That runs in a browser and makes web pages interactive. Also, it is a cross-platform, object-oriented programming language
What is the meaning of high-level programming language?
It means the language is softly coded. You don’t need to know all about your machine.
In other languages, you must know how the machine works, how the code will be compiled, how to run the code, and how the code will take the memory. But you don’t need to know all of this in a high-level programming language
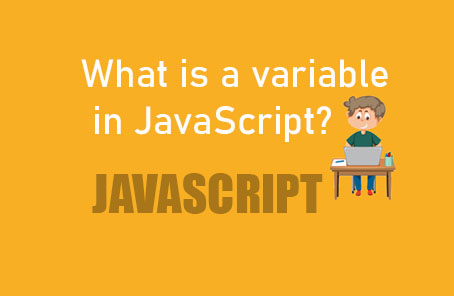
2 What is a variable in JavaScript?
The variable is like a container where you can store something. In JavaScript something means, you can say a lot. You can save numbers, strings, objects, even functions in a variable. A variable is like, suppose you’re saying ‘David is an honest person he is working on the farm’. Here, you see, first, you mentioned David’s name but then you didn’t mention the second time, it became ‘he’. This variable is similar to this. For example
3 What are the data types in JavaScript?
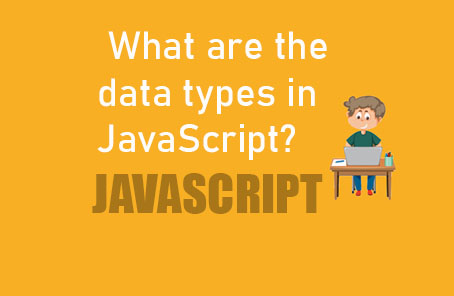
In JavaScript, there are primarily two types of data:
1 . Primitive data type
2 . Non-primitive data type
Primitive data type:
In primitive types, values are directly stored. In JavaScript, everything is essentially an object, but we’ll learn about objects later. Except for these primitive data types, everything else is not an object. For example:
4 What are the Operators of JavaScript ?
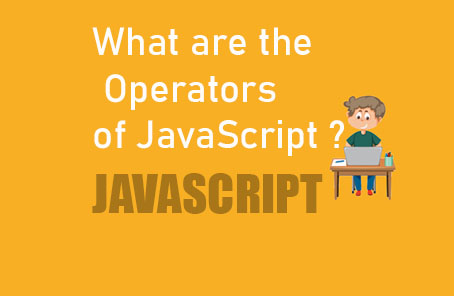
Let’s say 2 + 4 equals 6, where + is the operator, and 2 and 4 are the operands. Now, I will discuss this type of operator.
At this point, there might be a lot of confusion about what could be done with this. Doubts may arise, but these doubts are the basis of everything for you. So, you need to have a clear understanding of these. There’s nothing you need to remember or memorize. Let it slowly sink into your mind.
5 More JavaScript Operator
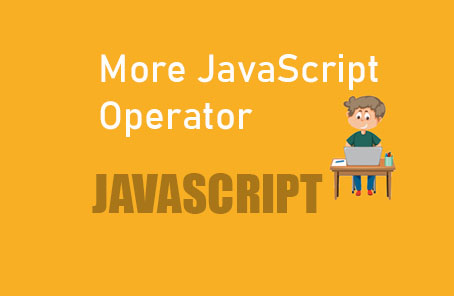
== , != , < , > , <= , >= Outside of these, there are a few more operators that are most used. For instance, === and !==. Pay attention, here there are three of them. They basically work like the basic equal == or not equal != operators. However, the real difference is that we’ve mentioned earlier that when comparing, the types of the two operands are sometimes converted to match each other if they are different types. In such cases, this part is skipped in the case of the triple equal, whereas with double equal, if the types are different, it changes the type to see if they are equal. Since types are changed under special rules here, developers might face problems with their usage initially. That’s why triple equal is more popular among everyone. With triple equal, your code will compare the values exactly as you provide them. There won’t be any type of conversion
6 What is a conditional statement in JavaScript?
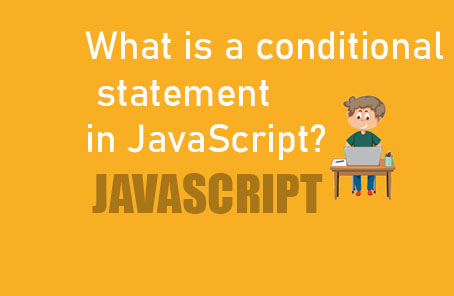
We make many decisions in real life. If today is Friday, there is no work, but if it’s Monday, there is an office or school/college. When we go to do something, like shopping in the market or various other ways, we have to make decisions.
In our programming as well, sometimes we have to make decisions like this. Suppose you have a website where users can register. On the other hand, many can visit without registering, whom we can call guest users or visitors. Here, if a user is registered on your website, you will show them one thing, and if not registered, you will show them something else.
7 What are the Loops in JavaScript ?
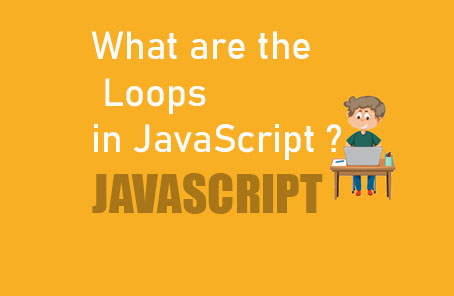
Sometimes in programming, we have to do a lot of repetitive tasks. In that case, we use loops. Here we will discuss various types of loops.
Loops are a very interesting thing in programming. To understand the concept of loops, we need to go a little into nature. In our world, there is day and night all the time. After a certain time, it becomes day again, and after a certain time, it becomes night again. This is a cycle, meaning a loop. In programming or in the tasks for which we use programming, there can be many tasks that follow such cyclical patterns.
8 Array in javaScript
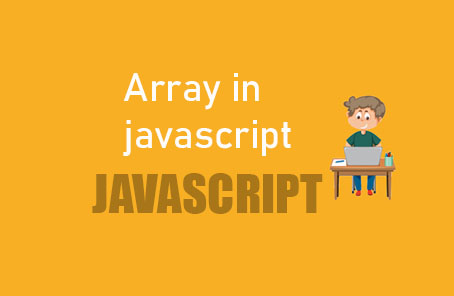
You want to store a lot of similar data. What can you do? You can store each separately with a variable for each.
Var name1 = “zonayed ahmed” ;
Var name2 = “jarif ahmed” ;
Var name3 = “david ahmed” ;
Var name4 = “masod ahmed” ;
9 What is an example of an object?
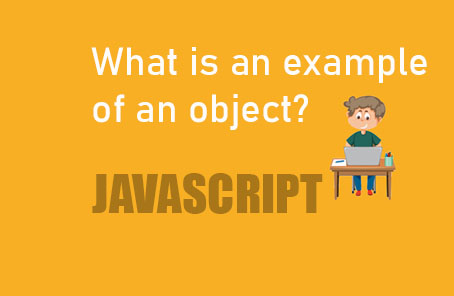
We have discussed arrays. Items in an array are accessed using index numbers. For this reason, the serial numbers of the items in the array must be kept in order. But if we want to access items from a data structure like an array using a specific key without an index, there is a way. That is using objects. Using objects is very handy and even more flexible than arrays. Suppose you want to store some information about a person. Now the pieces of information are somehow related to each other. In that case, we can use separate variables instead of an array. But even then, there is a problem with arrays. When we want to access items in an array, we have to do it by index number, which is not very meaningful. But what if something like that happens? How does it work?
10 What is the Function in Javascript ?
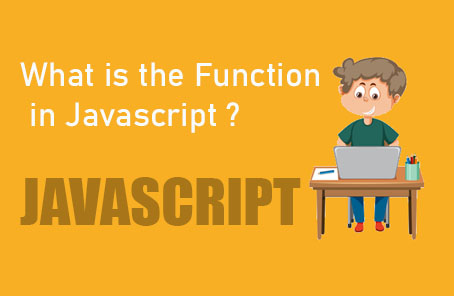
Suppose you want to know the result of 2 + 2. You write a few lines of code for that, and the result comes out correctly. Now, if you want to know what 4 + 4 equals, what will you do? Will you write a few more lines of code again? But, if you want to repeatedly add two digits each time, is there a way to organize it so that whenever I input two numbers, it gives me their sum? Ah, that’s where programming functions come into play. Today, I’ll talk to you about this function
11 What is a conditional statement in JavaScript?
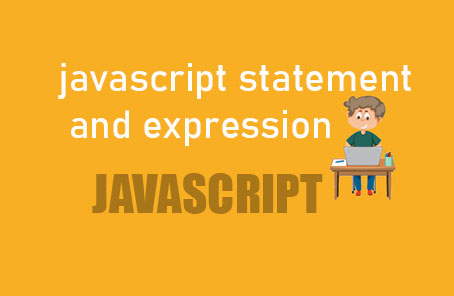
We make many decisions in real life. If today is Friday, there is no work, but if it’s Monday, there is an office or school/college. When we go to do something, like shopping in the market or various other ways, we have to make decisions.
In our programming as well, sometimes we have to make decisions like this. Suppose you have a website where users can register. On the other hand, many can visit without registering, whom we can call guest users or visitors. Here, if a user is registered on your website, you will show them one thing, and if not registered, you will show them something else.
12 DRY principle in javaScript
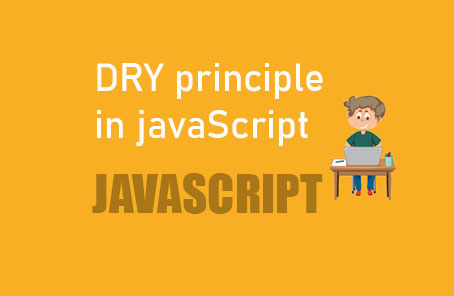
The ‘DRY’ principle, or ‘Don’t Repeat Yourself’, aims to shorten and beautify the process of not doing the same task repeatedly. In programming, situations may arise where we need to write code repeatedly to solve such problems. In such cases, instead of writing the same code repeatedly, we can use certain techniques to make one code do all the work. Additionally, the more lines of code there are, the more difficult it becomes to maintain. Finding problems can also take a lot of time. Therefore, there is no alternative to keeping the code short and beautiful to adhere to the DRY principle.
13 javaScript Behind the scenes

Coding is quite easy.But writing good code and understanding how that code works is much harder. I don’t mean to discourage you because it’s hard. Actually, understanding how the code works is the main thing. Once you understand how the code works behind the scenes, you can write code in any way that suits you. JavaScript typically runs in any environment. Most often, it could be our widely used browser or another application program like Node.js. Now, wherever this JavaScript runs, there is a JavaScript engine (Google V8 Engine, Spider Monkey, JavaScript Core, etc.), whose job is to execute this JavaScript code. Browsers use different engines to run JavaScript. For example, Google Chrome uses the Google V8 engine, Firefox uses Spider Monkey/Rhino, Internet Explorer and Edge use the Chakra engine, and Safari uses JavaScript Core/Nitro. There are many JavaScript engines, and for a full reference, you can visit www.web-w3.com. Now, this JavaScript code gets executed and runs in several steps. We can see this from the following
14 HOSTING IN JAVASCRIPT
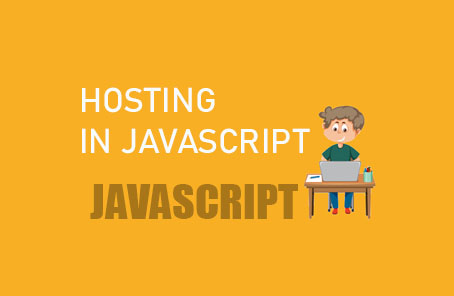
Actually, we don’t need to do anything about hoisting. It’s a default behaviour of JavaScript. I have previously discussed the “behind the scenes” of JavaScript. There, you may have seen that JavaScript brings all declarations to the top before anything else. This is essentially hoisting.
Since hoisting is a default behavior, all functions in JavaScript are hoisted during the creation phase. Therefore, we can call a function even before declaring it.
15 What is Scope in javaScript ?
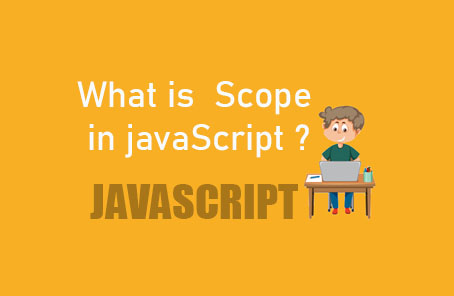
Scoping is another important topic in JavaScript. You need to know where you can access or use your declared variables/functions. If you want to make a variable or function private or accessible from everywhere, you need to know how and where to declare it, which will be discussed within scoping.
16 What is Closures in javaScript ?
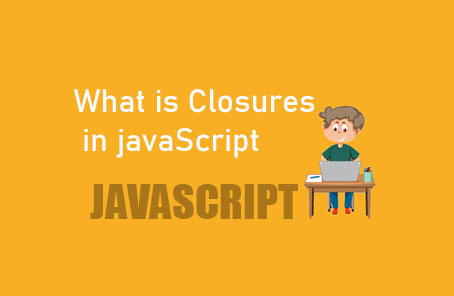
Closures are another topic related to lexical scoping. With closures, we don’t need to do anything separately; rather, it is JavaScript’s default behavior. However, understanding closures is essential for various techniques and to grasp JavaScript’s many surprising behaviors and specialties.
17 What is IIFE in javascript ?
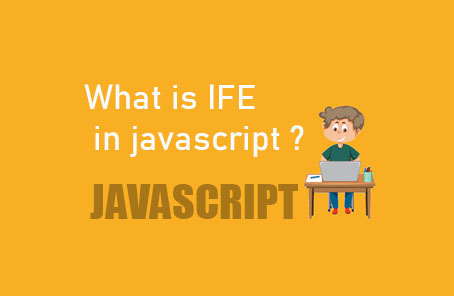
(Immediately Invoked Function Expressions)**
Usually, after creating a function, we need to call it to use it. But if we want to call the function immediately after creating it, we can use the IIFE (Immediately Invoked Function Expressions) technique.
In JavaScript, we can create a function in several ways:
18 JavaScript Advanced: The ‘this’ Keyword
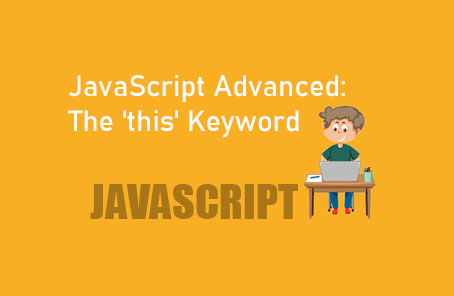
One of the trickiest and most confusing topics or keywords in JavaScript is ‘this’. It requires a deep understanding. However, in the beginning, you might have to use ‘this’ in your code with a fifty-fifty chance of getting it right. After practising several times and learning the rules, you will gradually understand the ‘this’ keyword completely. ‘this’ is a reserved keyword in JavaScript, meaning you cannot use this name for any variables or functions. It is used in many different ways and contexts. So, it’s not expected that you will understand ‘this’ in all situations from the beginning. But if you know the basic rules of how ‘this’ works, it won’t take long to understand even bigger and more complex code. The value of ‘this’ is determined based on how the function is called. Its value is set at the time of execution. Confusing? Yes, let’s start from scratch. Here, we will try to understand it in our own way. Just remember the following five rules that will help determine the value of ‘this’:
19 Understanding `call()`, `bind()`, and `apply()` Methods in JavaScript
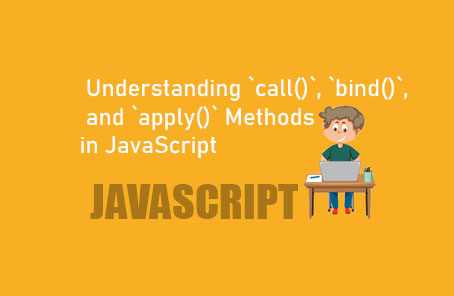
The this
keyword’s value or context can be determined using four rules, one of which involves explicit rules with call()
, bind()
, and apply()
. All three methods take the this
context or value as the first argument. Among them, call()
and bind()
can accept unlimited arguments, while apply()
accepts only two arguments: the first to set the value of this
, and the second, an array, to pass the function’s arguments.
20 Advanced Object in JavaScript
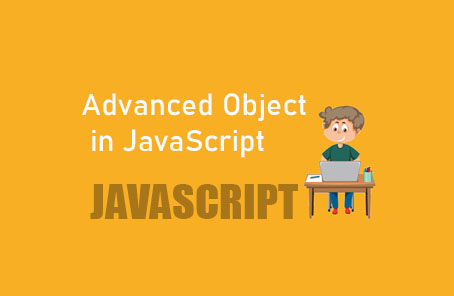
JavaScript is actually a prototype-based object-oriented programming language. As a result, in some cases, many things in JavaScript are a bit different from other OOP (Object-Oriented Programming) languages.
In JavaScript, almost everything is an object, except for some, which are called primitive data types. Based on this, there are generally two types of data types in JavaScript:
21 What is Function Constructor and ‘new’ Keyword in JavaScript ?
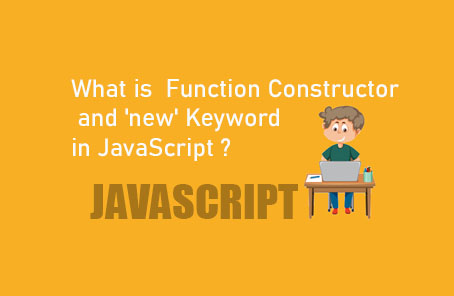
JavaScript is an object-oriented programming language, but those coming from other languages might miss one thing here: the class concept. Despite JavaScript being object-oriented, it operates differently compared to languages like C++ or Java, which are class-based. JavaScript is primarily a prototype-based language. In other languages, everything is encapsulated within classes, but in JavaScript, an object links to another object through a prototype. We previously discussed the prototype chain where objects are linked until the chain ends with null
, which has no prototype and marks the end of the chain.
22 What is Inheritance in Object-Oriented Programming (OOP):in javascript
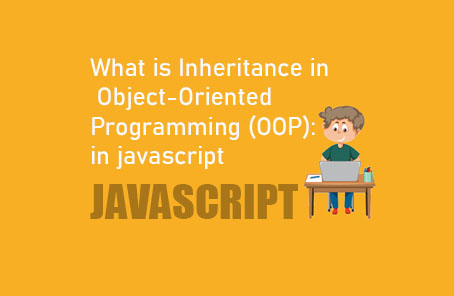
Inheritance is a very important and useful concept in object-oriented programming. With its help, features of one object can be easily taken into another object. Though it might not be clear here, you will understand it properly with a real example.
Suppose, we have a constructor function named `Person`. Now, what might a person have? A name, age, job, etc. So, if we create a blueprint for this, it would look like this:
23 What is Strict Mode in JavaScript?
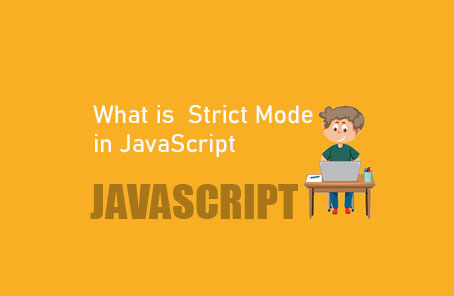
JavaScript sometimes exhibits unexpected behaviour, and to address these issues, the concept of Strict Mode was introduced to the developer community. In contrast, the non-strict mode is often referred to as Sloppy Mode. By default, if we don’t specify strict mode, the code operates in sloppy mode.
24 JavaScript ES6: What’s New?
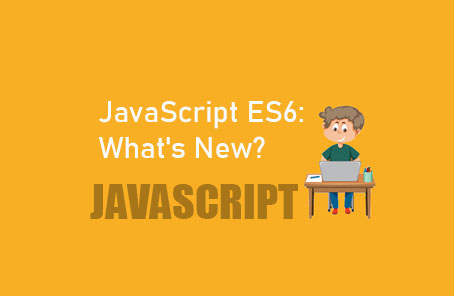
We have touched upon ECMAScript in our basic chapters. JavaScript has several versions, and in this chapter, we will discuss ES6 or ECMAScript 6. We’ll explore what’s new in it. But first, let’s look at the current state of JavaScript. There are several versions of JavaScript currently available:
– **ES5 (ECMAScript 5)**: This is the fully supported version of JavaScript in all modern browsers and environments. You can start using ES5 without any hesitation because it’s universally supported, so there’s no need to worry about compatibility.
25 Variable Declaration with `let` and `const` in JavaScript
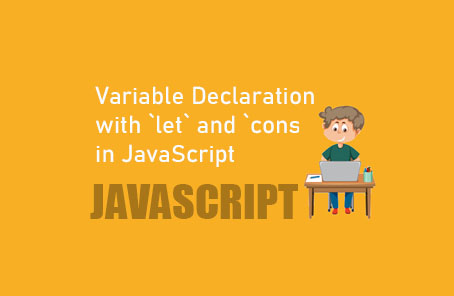
In JavaScript, traditionally, all types of data were declared using the `var` keyword. Due to its high-level nature, you don’t need to specify whether the data type is an integer, character, or string; JavaScript determines it automatically. By using `var`, you can declare variables without hassle.
However, ES6 introduced two new keywords: `let` and `const`. These keywords do not have any relationship with data types, just like `var`. You can use them to declare any type of data. The main difference lies in their behaviour.
26 Explanation of Immediately Invoked Function Expressions (IIFE)
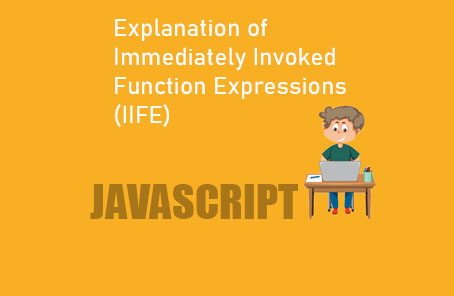
(Immediately Invoked Function Expressions)**
Usually, after creating a function, we need to call it to use it. But if we want to call the function immediately after creating it, we can use the IIFE (Immediately Invoked Function Expressions) technique.
In JavaScript, we can create a function in several ways:
27 What is Template Literals in javascript ?
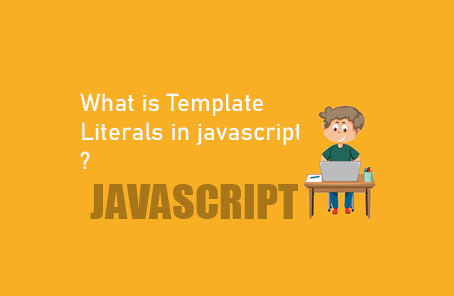
In JavaScript, if we want to print a string along with some variables, we usually have to concatenate those variables using the +
operator. This process can be quite tedious, especially for long strings, where it’s easy to get lost. For instance, let’s say I want to print some information from a few variables:
28 Advance javascript String Methods
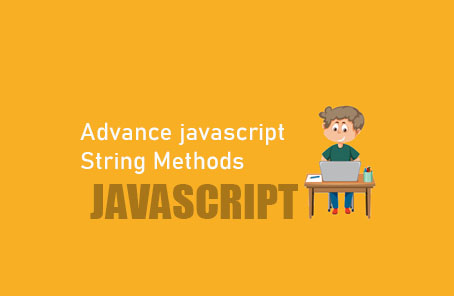
String Methods
With ES6, some new string methods have been introduced. Here, I will discuss some very useful methods among them.
– To check if a string starts with a specific character/string, use the `startsWith` method.
– To check if a string ends with a specific character/string, use the `endsWith` method.
– To check if a string contains a specific character/string, use the `includes` method.
29 What is Arrow Functions in javascript ?
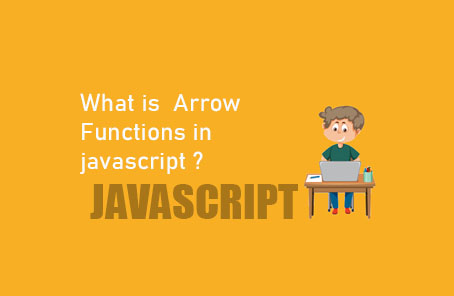
A new addition to JavaScript’s ES6 is the arrow function. Many also refer to it as the fat arrow function. It’s not actually something new, but rather syntactically pleasing and clean. In the programming world, there’s a term called “syntactic sugar.” It means syntax that looks nice and feels clean. Although the functionality of arrow functions is quite similar to ES5 functions, there are some differences.
30 Lexical ‘this’ Keyword in javaScript
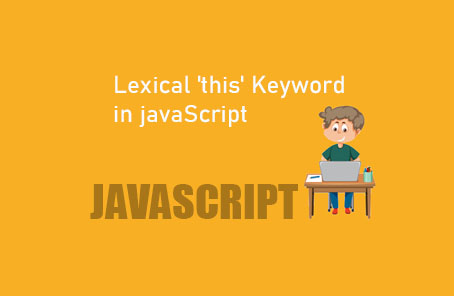
Lexical ‘this’ Keyword
So far, what we have discussed are mostly syntactic sugars. The biggest difference between arrow functions and normal functions lies in the `this` keyword. We know that in ES5, the value of `this` in a function depends a lot on how the function is called. Each defined function in ES5 influences the value of `this`. However, the ES6 arrow function does not have its own impact on the `this` keyword. Instead, it takes the `this` value from its surrounding context.
31 What is Destructuring in javascript?
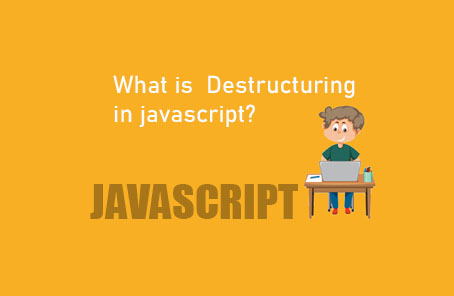
JavaScript ES6 and later versions are primarily designed for smart programming. By using ES6, you will become more efficient, enabling you to do more with less code. Destructuring serves this purpose. It is not a new feature but rather a technique, a smart programming syntactic sugar.
32 JavaScript Code of es6 advance array
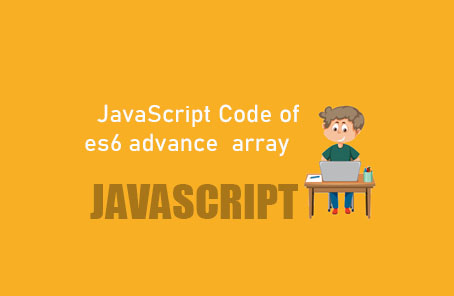
Arrays are nothing new in JavaScript. But ES6 introduces some new array methods. As mentioned earlier, ES6 comes with new techniques designed to make our lives easier. To simplify our lives, powerful new methods have been introduced.
33 What is . Spread Operator in javascript ?
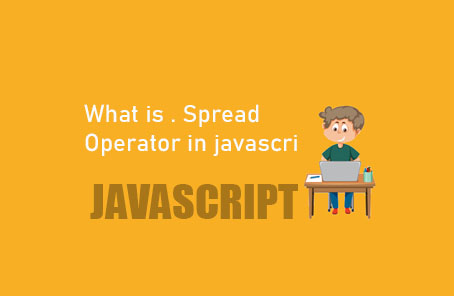
The spread operator is another smart feature in JavaScript ES6. Many people hesitate to use it because it seems complicated. However, after paying a little attention or using it a few times, you’ll realize how useful it is. In truth, it’s also a kind of syntactic sugar.
The main function of the spread operator is to spread iterable data, such as arrays or strings, into their individual elements. It does exactly what the word “spread” means in English. The spread operator is essentially three dots. These three dots can be used in other contexts as well, which we will discuss later. For now, let’s look at an example to understand what the spread operator does. First, let’s see a program in ES5 version.
34 What is . Rest Parameter in javascript ?
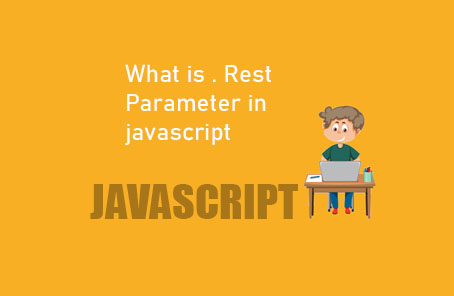
The rest parameter allows you to pass any number of parameters into a function. When we define a function with parameters, if it is unknown how many arguments might be passed, we use the rest parameter or the arguments object from ES5. The arguments object in JavaScript ES5 essentially did the same job, but the rest parameter in ES6 is a bit smarter. The rest parameter uses the same three dots (ellipsis) as the spread operator. Therefore, you need to understand when these dots are used as a rest parameter and when they are used as a spread operator.
35 What is . Default Parameters in javascript ?
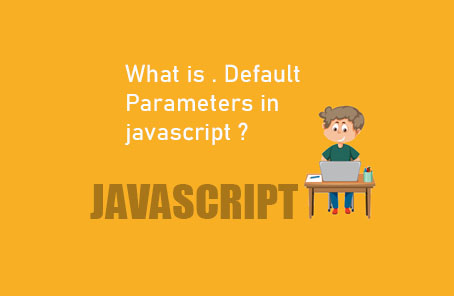
JavaScript introduced ES6 primarily to make our lives easier. One of the additions to achieve this is the default parameter feature. Many people expected such behavior from JavaScript earlier, and that’s why the default parameter was added.
If we want to set a default value for a parameter while defining a function, we can easily use ES6’s default parameters. However, in ES5, it wasn’t as straightforward. You can understand this by looking at the following examples.
36 Advance Map in javascript es6
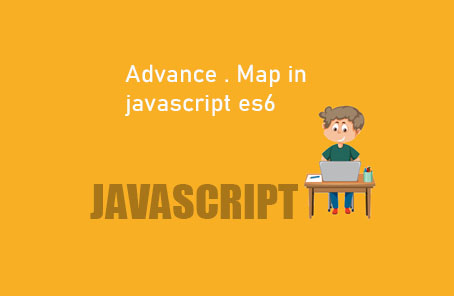
In JavaScript’s ES6, apart from some syntactic sugar, we haven’t seen anything significantly new so far. But now we are going to discuss something entirely new. Yes, this feature has been added completely new in ES6. It is called Map.
37 What is Advance Class in javascript?
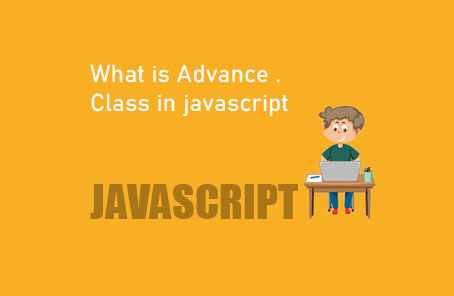
Until ES5, JavaScript did not have the concept of a class, unlike other programming languages. Those who come to JavaScript from other programming languages often get confused due to the absence of this feature and question whether JavaScript truly qualifies as an object-oriented programming language.
38 What is Class Inheritance in javascript ?
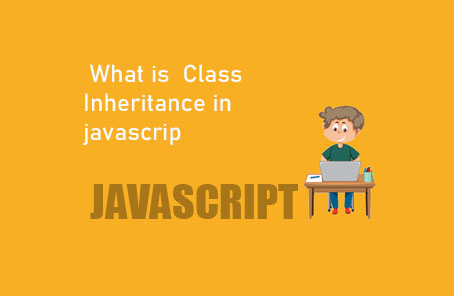
Now, if we encounter a situation where we may have two or more classes that are similar, as seen even in ES5 constructor functions, we can utilize inheritance. For example, let’s say we have a class called PersonClassDemo
:
39 All about es6
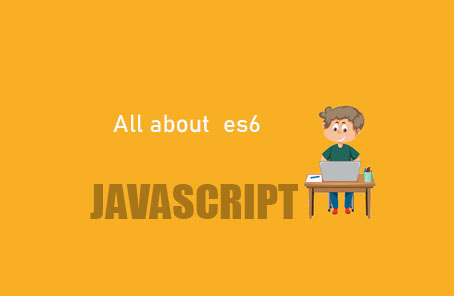
In all environments, ES6, the new version of JavaScript, allows us to write code more simply and elegantly. Compared to ES5, ES6 often requires less code and solves many problems with fewer lines of code. It looks nice, involves fewer lines of code, and is smarter in every way. So, should we still use ES5 or switch to ES6? From one perspective, due to the new syntax and many other advantages of ES6, it might be wise to use ES6. Additionally, as developers, we don’t like to stay outdated. Therefore, if we want to stay updated, we should start using ES6 now.
40 First Class Functions and Higher Order Functions in javascript
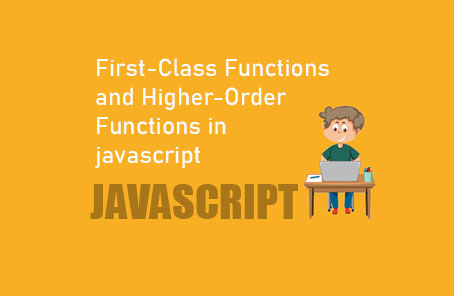
In JavaScript, functions are called first-class functions. So, what is a first-class function? A first-class function can be passed as an argument to another function, a function can return another function, and most interestingly, a function can be stored as a variable.
41 The some() and every() methods in javascript
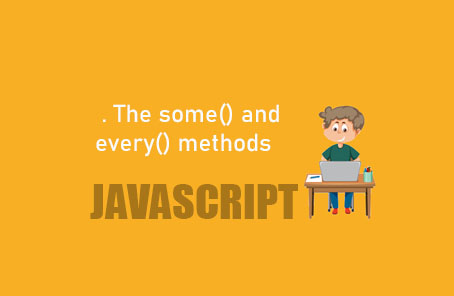
are generally used when there is multiple data in an array. These methods help us filter or operate on data within the array as needed. Similar to the previously discussed map, filter, and reduce methods, there are two more useful methods: some() and every(). These methods share similarities with the earlier map and filter methods. We might encounter situations where we need to check if a specific data is present in an array. For instance, we have an array like this: const arr = [1, 2, 4, 5, 6, 7, 8]; Now, we want to know if this array contains the number 7. Or, we
42 How to Run loop on object in javascript ?
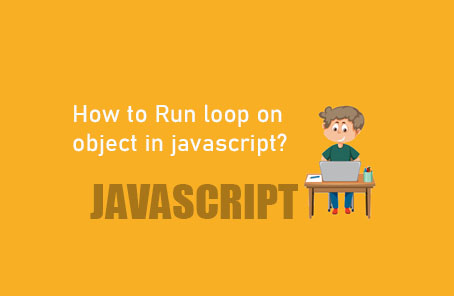
Although everything can be done this way, JavaScript developers prefer functional programming. However, if we need to take all values or an array, it may cause a little trouble here.
I now want to print the capitals of all countries. Now we can see the methods of the Object constructor here. Each object created here has an Object constructor.
43 What is Dot Notation and Brackets Notation in javascript ?
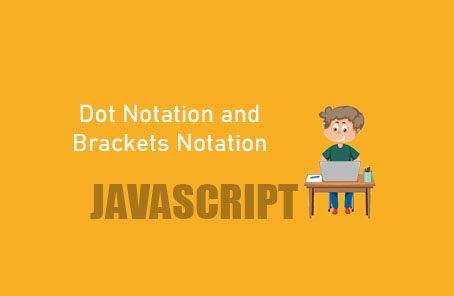
Now, if both give the same output, what is the need for having both? Wouldn’t one be enough? But no! While dot notation is used most of the time, there are situations where brackets notation is also useful. However, one thing to note is that where dot notation works, it is conventionally preferred (a favorite among developers).
44 What is Logical Operators OR (||) in javascript ?
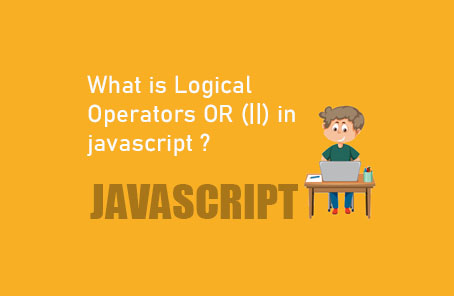
We learned about logical operators in the basics of JavaScript. Here, we will discuss two logical operators: OR (||) and AND (&&). Although they seem simple, they can perform much more complex tasks. Let’s delve into their usage.
Before we start with these, let’s discuss another aspect of JavaScript. In JavaScript, except for some special values, everything else (expressions) is considered a true expression. What does this mean? Let’s see the example below:
45 What is Logical Operators and (&&) in javascript ?
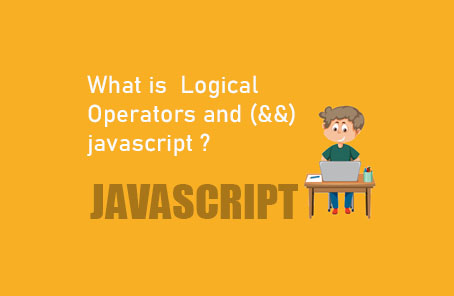
This && operator is used between two expressions:
‘Go to the market’ && ‘Do the shopping’
Now, notice that you can only do the shopping if you go to the market: ‘Go to the market’ and ‘Do the shopping’.
The AND operator works in exactly the same way. If your first expression is true, only then will the second one execute.
And if the first one is false, then the second one will never execute:
46 The conditional or ternary operator in javascript
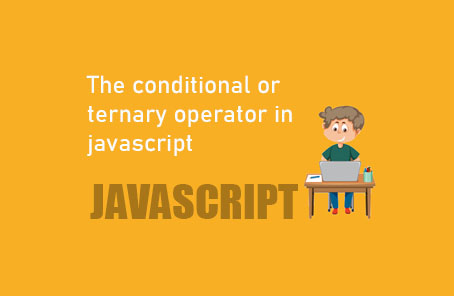
is often used as a shortcut instead of if-else statements in many cases. In this operator, a decision based on a condition can be made in one line. It works somewhat like this: condition ? this : that
. Here, these two special things are used. Suppose we have a variable below: const age = 21;
. Now, based on this age
, we want to print a message. If the age is below 18, we want to print one message, and if it’s 18 or above, another message. We can do this using if-else like this:
47 Regular Expressions in javascript
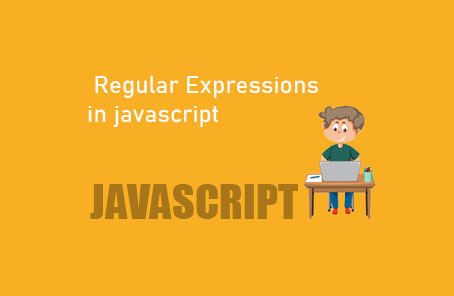
Regular expressions are a common concept in all programming languages. Although there might be slight differences between languages, the core idea remains the same. A regular expression is a sequence of characters and symbols that allows you to search for a specific pattern in text or strings and take actions based on that.
48 Error Handling in javascript
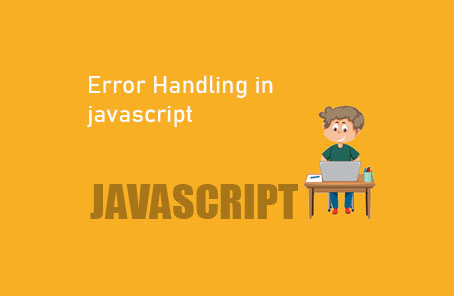
Errors are our everyday companions. If you write code, there will be errors. However, many of us think that errors are just errors, and we have no control over them. Yes, this is partially true but not entirely. Additionally, a good application should ensure that users are unaware of any errors. Users will not open the developer console like us to see if there is an error, so we need to incorporate the errors into a good user experience. This is why error handling is necessary. We will discuss this here.
49 Callback Function in javascript
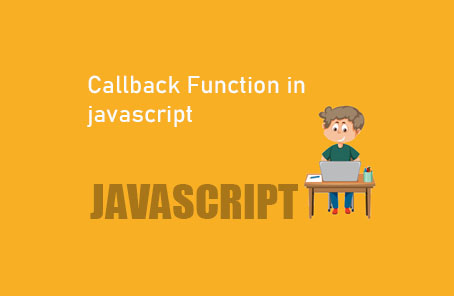
What is a callback function? You might have heard about it in many places. But what exactly is a callback function? Yes, in short, a callback function is a function that is sent as an argument to another function. And it is called from that function.
We know about higher-order functions in JavaScript. Here, functions are also objects. One function can return another function, and at the same time, a function can take another function as an argument. And whenever a function takes another function as an argument, we call that argument function a callback function.
So, what does a callback do? We know about JavaScript’s asynchronous behavior: