What are the data types in JavaScript?
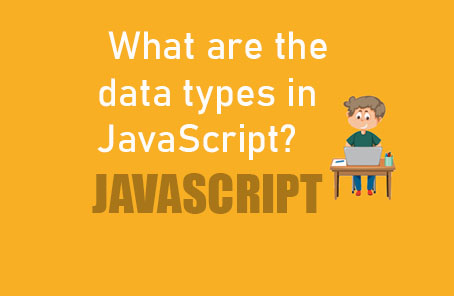
In JavaScript, there are primarily two types of data:
1 . Primitive data type
2 . Non-primitive data type
Primitive data type:
In primitive types, values are directly stored. In JavaScript, everything is essentially an object, but we’ll learn about objects later. Except for these primitive data types, everything else is not an object. For example:
( 1 ) Number: It can be any type of number, starting from a normal number. It can also be a decimal. For example –
Var aNumber = 10 ;
Var anotherNumber = 10.50 ;
( 2 ) String: text with a Number, means a sequence of characters with text and a Number inside. The sorting must be within single quotes (‘ ‘) or double quotes (” “), as per your preference. Using double quotes once and single quotes once is not correct, so wherever you start writing, you will continue in that manner. If you write a number within double or single quotes, it will also be considered a string. For example
Var text = ‘ I want to say something ‘
Var isString = “10” – // it also a string type //
( 3 ) Boolean: This type can have two values. True or False, all lowercase.
An uppercase value will be considered incorrect. There are no “ “ or ‘ ‘ in boolean
For example
Var isTrue = true ;
Var isFalse = false ;
( 3 ) Undefined : When you declare a variable but do not assign any data to it, then the value of that variable remains default undefined
Var something ;
( 4 ) null : This has no existence. But it’s not undefined either. It means you don’t want to store anything in your variable, but you also don’t want it to be undefined. The correct spelling of null will be null. Null or NULL is wrong.
Var someThing = null ;
Non-primitive data type :
In non-primitive data types, values are not directly stored. Instead, references to values are stored, and these types hold data objects. This means they have many properties. Examples include:
- Array
- Object
- Function
We will discuss non-primitive data types in detail later, as each of them warrants detailed discussion. These are objects, each with its own properties. Therefore, we will discuss each aspect separately as we progress further.
Concatenation :
Now, let’s talk about a few more basic topics regarding concatenation or adding multiple data together in JavaScript, which is done using ‘ + ‘
Var text = “how” + “are you” ;
Var welcomeMsg = “Hello” + text ;
console.log(welcomeMsg)
The result will : Hello how are you
If you want, you can store the entire add as a variable. However, we know that in JavaScript, everything is declared using the ‘var’ keyword. Now, if we store everything in a single variable, what type will it be? Yes, this is why in JavaScript, the typeof operator is used to check the data type. For example, if we want to see the type of a variable called ‘welcomeMsg’ that we used earlier:
typeof(welcomeMsg) = “String”
If you add several types together and save them in a variable, the type of the variable will depend on your data. If you add a number to another number, it will be addition, not a concatenation. And if you add a number to a string or a string to a number, then it will entirely become a string type because numbers cannot be saved without numbers. However, numbers can be saved with string characters, as here, where it will be addition.
Var number = 10 + 10 ;
console.log(number)
Output: 20
Now if you check the type of this number variable
typeof(number)
The output will “number”
Now for a string
Var isString = “ Hello there + 20”
console.log(isString)
Output will “Hello there20”
Now if you want to check the type of isString
typeof(isString)
“String”
Post Comment