What is a conditional statement in JavaScript?
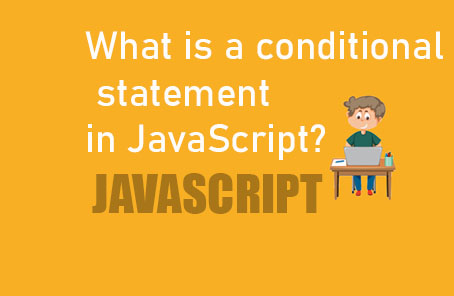
We make many decisions in real life. If today is Friday, there is no work, but if it’s Monday, there is an office or school/college. When we go to do something, like shopping in the market or various other ways, we have to make decisions.
In our programming as well, sometimes we have to make decisions like this. Suppose you have a website where users can register. On the other hand, many can visit without registering, whom we can call guest users or visitors. Here, if a user is registered on your website, you will show them one thing, and if not registered, you will show them something else.
In the example of Facebook, if you don’t register/login, you’ll see a login/registration page. And if you register and login, you’ll see something different. So, these are actually decisions in programming. These are controlled by conditional statements.
In JavaScript, there are three types of conditional statements.
- If ( statement)
- If …..else ( statement )
- If…else if ( statement )
There’s also another one called the switch statement, which is particularly useful in many cases. So, discussion on that topic has also been included towards the end of this topic.
Here, we’ll need to write multiple lines of code, and for that, you can use the console.
The real job of a conditional statement is that if any statement is true, then only the code/program inside that conditional statement will run. And if it’s not true, it won’t execute any part of that code.
1.if statement
if( anything is true ) {
So, the code inside will execute.
}
It will only check a condition. If it’s true, then what’s inside will be visible. Otherwise, there’s no need to show anything.
if( 3 < 5 ) {
console.log( “yeah i will be executed”) ;
}
> Yeah i will be executed.
Comparing the two numbers will result in true/false. And based on that, whether the console.log inside will print or not will depend. Since 3 is less than 5, the expression is true, and the console.log inside will be printed.
More like this :
Var myName = “Zonayed” ;
if( myName === “Zonayed” ){
console.log(“you are allowed”)
}
“The strings are compared in the same way since the two strings are the same, so the text has been printed.
Var myName = “Zonayed” ;
if( myName === “karim” ){
console.log(“you are allowed”) }
This expression is false, so nothing gets printed.
You can write it repeatedly like this.
Var myAge = 20 ;
If ( 9 > 10 ) {
console.log(“ good to go” ); }
If (myAge >= 20 ) {
console.log( “ You are adult” ) ; }
if( myAge < 20 ) {
console.log(“ you are not adult “ ); }
Output: you are adult !
Expressions that evaluate to true have been logged or printed inside their console. However, if conditions occur based on a subject or a cause, then it’s best to use the ‘if…else if’ structure. And you must remember, in ‘if (expression)’ here, the expression must evaluate to true, otherwise, it will result in false. Conditional statements only accept true or false, nothing else. Do you remember from the previous topic how operators show true or false results? Yes, you can use those here as you wish.
Var myAge = 21 ;
if(myAge > 20 && myAge < 60) {
console.log( “you are a young person “ ) ;
}
You are a young person
2. if … else statement
if( anything true ) {
This code will executed
}
Else {
This code will executed
}
This is stating that if the condition within the ‘if’ statement evaluates to true, it will execute what’s inside the ‘if’, otherwise it will execute what’s inside the ‘else’. It gives you more control over conditional statements. Here, if the condition is true, the code inside the ‘if’ will execute. And if it’s false, the code inside the ‘else’ will execute.
Var age = 21 ;
if( age > 18 ){
console.log( “now you are adult” ) }
Else { console.log( “you are not adult” ) }
The truth is, this is printed, and if it’s false, that will be printed. Here, the truth has been printed.
Output: now you are adult.
3. If….else if Statment :
if ( is anything true ) {
This code will executed }
Else if (or If I am truthful ) {
Then this code will executed }
Else if ( or if I am truthful ) {
Then this code will executed }
Else { this code will be executed }
Here… meaning, as many times as you wish, else if can be used. This means, if some truth exists, it will show what’s inside me; otherwise, if something else is true, it will show those, or if nothing is true, else will show what’s inside me.
Nesting conditional statements :
Nesting means having one conditional statement inside another conditional statement, and it is completely valid. You can use else if…else within if, or else if within others, or just if within others. There are no restrictions or rules here.
Var num = 10 ;
If ( num > 1 ) {
if(num > 10 ) {
console.log(“Greater then 10”) ;}
Else{ console.log( “somewhire between 2 – 10 “ ) ; }
}
You can have one inside another, another inside one, as many times as you want.
Switch Statement
Now let’s talk about the switch statement. What we used at the end, if…else if, may not always be flexible. Suppose you want to make a decision on top of a pile of sand. If the case is smooth, it will print one thing, and if the case is rough, it will print another.
An example :
Var weekDay = “sunday” ;
Switch (weekDay) {
Case “Saturday” ;
console.log(“saturday ! today is closed” ) ;
Break ;
Case “sunday” ;
console.log(“Sunday ! it will normal day” ) ;
Break ;
Case “friday” ;
console.log(“friday” ! today is closed “ ) ;
Break ;
Default ;
Console.log (“Not a day”) ;
}
Wow! That’s a lot of code, but it’s quite simple. See, although switch is not the best solution for this task, you can use whatever you like. Here, in every case, you’ll see ‘break;’ written. If you don’t write this, the code will continue running until the very end from the case where your value matches. ‘break’ indicates that the basic task is done, stop now. And at the end, see there’s a default case, if none matches, this will be printed. Whatever case matches, that’s what gets printed.
>>> Sunday ! it is normal day
Post Comment