What are the Loops in JavaScript ?
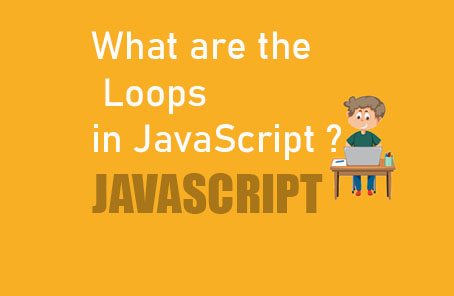
Sometimes in programming, we have to do a lot of repetitive tasks. In that case, we use loops. Here we will discuss various types of loops.
Loops are a very interesting thing in programming. To understand the concept of loops, we need to go a little into nature. In our world, there is day and night all the time. After a certain time, it becomes day again, and after a certain time, it becomes night again. This is a cycle, meaning a loop. In programming or in the tasks for which we use programming, there can be many tasks that follow such cyclical patterns.
The time of a clock can be an example too. Even if you start the timer, its value will keep increasing until you stop it. This can be described in this way.
“As long as (it is not turned off)
the clock will keep running.”
Or suppose you want to move your character in a game. Now you tell your character to move ten steps forward from a certain position. Steps range from 0 to 10, with one foot per step moving forward
In programming, loops come in many forms. However, in JavaScript, there are several types of loops commonly used. Below are the statements used for loops:
- For statements
- Do…while statements
- While statements
- Break statements
- Continue statements
Although loops are not extensively used in JavaScript, since they are common concepts in most programming languages, it’s essential to have a good understanding of them. Then you’ll be able to grasp any concept later easily. They are essentially the base. If you have a strong base, you’ll be able to learn anything later effortlessly.
- For statements:
In JavaScript, the ‘for’ loop functions similarly to other common programming languages. Typically, the ‘for’ loop is used when you want to perform a certain task for a specified period of time. Here, you will specify the starting point, then determine how long it will run, and finally, indicate how much to increment at each step.
for (start; condition; step) {
statement
}
For example :
For ( var i = 0 ; i < 10 ; i++) {
Console.log ( “Go” + i + “step” ) ;
}
I have initially set the value of ‘i’ to 0 here and then set a condition that ‘i’ must be less than 10. If this condition is met, the statement inside will execute, otherwise, if it’s equal to or greater than 10, the statement inside will not execute, and it will exit the loop. Then, I have used ‘i++’, which means ‘i’ will increment by 1 in each step. You’ve seen the operator section here. And here, continuity must be maintained as per the given sequence
- Do… while statements :
It’s another loop, but if you can’t specify exactly when your loop might end, then this loop is used.
Do{
statements
}
As long as (I am true).
In this do while, your loop will run the inner code once without checking any condition, and then after that your condition will be checked. If the condition is true, then the things inside the loop will run again and it will continue like this until your condition becomes false. If the condition becomes false, the loop will stop.
Var num = 15 ;
Do { console.log(“ Inside the loop , num is: “ + num ) ;
Num += 1;
}
While (num < 10 );
console.log( “outside the loop” );
We want the loop to run as long as the value of num is less than 10. However, the value of num is 15 here. Still, the loop has run once because it runs once before checking any conditions. That’s why the code inside the loop ran once.
Inside the loop , num is: 15
Outside the loop
- While statements :
While (I am true) {
Statement;
}
This is just like another one, but first, it will verify your condition. If it is false, then it will immediately exit the loop without asking any questions or executing anything. And if it is true, it will execute from within the loop.
Var num = 10 ;
Do { console.log( “ inside the loop, num is: “ + num ) ;
Num += 1 ;
} while (num < 20 ) ;
console.log( “ outside the loop” ) ;
I want to run the loop as long as the value of num is decreasing from 20′. At the same time, within the loop, the value of num is incremented by 1 for each iteration, so that at some point its value becomes 20′. And if the expression inside the while loop becomes false, the loop terminates.
If I set the value of num to more than 10, then it will never execute anything inside the loop.
Var num = 20 ;
While ( num < 10 ) {
console.log(“inside the loop”) ;
Num += 1 ;
} console.log(“Out of the loop”)
Nothing will run inside the while loop.
>>> Out of the loop
If it’s a while and do…while loop, then at least once it will enter inside the loop.
- Break statements:
You can control the loop from within the loop’s statement. If you want to break out of a loop at a certain time during its execution, you can use the break statement.
For ( var i = 0 ; 1 < 10 ; i++ ) {
if( i === 5 ) {
Break ;
}
console.log(“ i is now at: “ + I ) ;
}
We have set a condition here that if the value of ‘i’ becomes 5, then break. So, whenever the value of ‘i’ becomes 5, the program will immediately exit the loop.
- Continue statements :
This is also used to control loops in this way. But in this case, instead of exiting the loop, the loop is simply skipped.
For ( var i = 0 ; i < 10 ; i++ ) {
If ( i === 5 ) {
console.log( i + “ is skipped” ) ;
Continue ;
}
Console.log ( “ i is now at: “ + i ) ;
}
We’ve added a condition here just like before. If the value of ‘i’ ever becomes 5, then print a message and continue this loop with the next iteration. And that’s how you’ll get the output in the same way.
Post Comment