What is an example of an object?
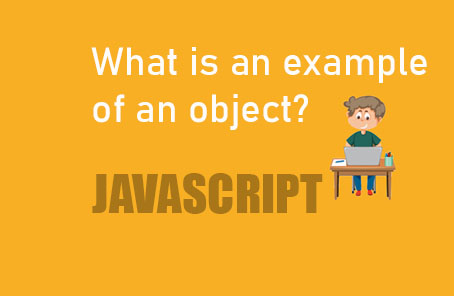
We have discussed arrays. Items in an array are accessed using index numbers. For this reason, the serial numbers of the items in the array must be kept in order. But if we want to access items from a data structure like an array using a specific key without an index, there is a way. That is using objects. Using objects is very handy and even more flexible than arrays. Suppose you want to store some information about a person. Now the pieces of information are somehow related to each other. In that case, we can use separate variables instead of an array. But even then, there is a problem with arrays. When we want to access items in an array, we have to do it by index number, which is not very meaningful. But what if something like that happens? How does it work?
aObject = {
Name: Jason,
Address: New York,
Old: 22 ,
}
Let’s say, aObject is an object, then its properties are name, address, age, etc. These properties are related to each other. In this case, this data structure works perfectly. And now, if you want to access the information or properties of aObject, like its name or age, then you can simply write the object’s name followed by the property you want to access. Yes, in JavaScript, objects work the same way. In this way, without using array-like indexes, you can use keys to access the items.
An example of an object
Var davide = {
fullName : “david jason” ,
age : 21 ,
Address : “new york” ,
Job: “job holder”
}
console.log(davide) ;
> var davide = {
fullName : “david jason” ,
age : 21 ,
Address : “new york” ,
Job: “job holder”
}
< undefined
> console.log(davide)
> { fullName : “david jason” , age : 21 , address : “dhaka” , job : “job holder” }
Now you can see the entire object here. Clicking on the triangle button on the left side will also reveal everything here. However, instead of index numbers, you can see keys, which you can use to access the items of this object.
Once again, how can we access objects? Although we discussed this in the previous section, it will be more useful here. We can access items from an object in two ways.
- Dot notation
- Bracket notation
Let’s now discuss these notations in detail.
- Dot notation
In JavaScript, accessing a property inside an object is commonly done with this notation. At the beginning, I mentioned that if we want to access the name of David, we just need to say ‘David’, and in JavaScript, this ‘of’ essentially indicates a dot (.). If we want to access the full name of David using dot notation, we just need to mention the object’s name followed by a dot and then the key of the full name.
davide.fullName
> davide jason
- Bracket notation
Again, bracket notation can also be used to access items in an object.
davide[“fullName”]
> davide
< {fullName : “david jason” , age : 21, address: “dhaka” , job: “job holder” }
> davide[“fullName”]
< “david jason”
When an object has been declared, if we want to add a new item here, is there a way? Ah, we’ve seen that the object doesn’t have any index numbers, so maintaining the serial number of each item here is not necessary. We can access any item using just the keyword. Once the object is declared, if we want to add a new item later:
davide.zipCode = 3517
{fullName : “david jason” , age : 21, address: “dhaka” , job: “job holder” , zipCode = 3517 }
Such a beautiful addition! Now, if we already have it, we want to change the value of that item.
Davide.job = “Student”
{fullName : “david jason” , age : 21, address: “dhaka” , job: “Student” , zipCode = 3517 }
Now, what’s even more special about objects is that you can store functions inside them as items. If you’re not sure what a function is, we’ll discuss it later. For now, just keep in mind that functions can be taken as items within an object.
davide.welcomeMsg = function () {
console.log(“holle world”) }
> davide
>{fullName : “david jason” , age : 21, address: “dhaka” , job: “Student” , zipCode = 3517 , welcomeMsg: f () }
If you want to access the same function, it needs to be done in the same way. Because when calling a function, the first bracket must be used.
davide.welcomeMsg()
“Now, when defining the above function as an object, I can take it like a variable in this way.
Var davide = {
fullName = “david jason”,
Age: 21 ,
Address: “dhaka”,
Job: “job holder “ ,
welcomeMsg: function() {
console.log(“hello there” ) ;
} }
In both cases, first we have declared the object, then assigned the function to it. And in the second case, while defining the object, we have given both function and variable like the other.
Array inside an object :
Var objArr = {
Normal : “normal item “ ,
Name: [ “Rahim” , “Karim” , “Rafiq” , “Shafiq” ]
}
The items inside, being arrays and objects, can be accessed following the rules of arrays and objects. Suppose I want to access Rafiq’s name.
objArr.name[2]
“Rafiq”
An object within the array:
We know that we can store many items in an array. Now those items can be another array, a number, a string, a function, a boolean, or another object.
Var arrObj = [
“Zonayed” ,
{ name : “rafiq” ,
Age : 21 ,
Job : “student “
} ,
“Bangladesh”
]
Here, an entire object will be counted as one element of the array. Now, can you tell me the index of the object? Yes, the index of the object is number 1, and then the index of Bangladesh is number 2 – and if you want to access items from this object.
In this way, you can take objects inside an array, then an array inside that object, and again an array inside the array, or an object inside an object within the array. And as long as you follow the rules of arrays within arrays and objects within objects, you can create nested data like this and access items from within.
Post Comment