What is the Function in Javascript ?
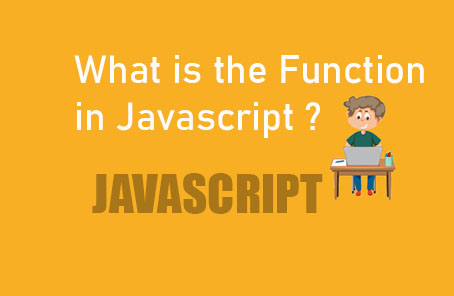
Suppose you want to know the result of 2 + 2. You write a few lines of code for that, and the result comes out correctly. Now, if you want to know what 4 + 4 equals, what will you do? Will you write a few more lines of code again? But, if you want to repeatedly add two digits each time, is there a way to organize it so that whenever I input two numbers, it gives me their sum? Ah, that’s where programming functions come into play. Today, I’ll talk to you about this function.
A function can be compared to a machine. Here you just provide some input and it gives you output according to your preference. Suppose you have some cloth, and you want to make a dress out of it. What will you do? Maybe you can make it yourself, or in most cases, what we do is take the cloth to a tailor shop and provide them with our body measurements. After a certain time, we go and pick up the dress. You don’t need to know what the tailor did with your clothes. You just gave the cloth, and they made the dress according to your preference.
If you give an example of the sum of two numbers in the same way
Addition_machine (number1 + number2 ) {
Addition = number1 + number2 ;
Return addition
}
Show addition_machine(2 , 2)
Show addition_machine (4 , 4 )
When we talk about JavaScript, we’ve learned that everything is an object. So, what are functions are objects? Yes, in JavaScript, functions are objects.. Functions in JavaScript are called first-class functions. Because, you can return a function from a function if you want, and you can also store a function in a variable. Again, you can pass another function as an argument to a function. I will discuss with examples below to make everything clear.
We’ve already used many built-in functions, such as console.log(something. here). Here, we’ve written console dot log and then something inside, and it got printed in the browser console. Now, we don’t really need to understand what console dot log does internally and how it prints in the browser console. Because, this too is a function. And its job is to print the input we provide to the browser. There are many more built-in functions like this that have already come with JavaScript. They start working as soon as you call them.
Now there may come a situation where we need to create our own functions instead of relying on built-in functions. In that case, we can create our own functions. Now let’s talk about how to declare a function. To declare a function, we always use the ‘function’ keyword. Then we need to give the name of the function, followed by curly braces { to start, and inside them, you’ll put what you want to do, those will be statements, and finally, it should end with curly braces }
Function funcName () {
// statement
}
Declaring a function like this is called a function statement. Again, functions can also be declared in this way
Var funcName = function () {
// statement
}
I have assigned any anonymous function here to a variable. Declaring a function like this is called a function expression. Now, let’s talk about declaring a function. So, how do we use it? Well, you have to call or invoke the function. If you don’t call it, the function won’t execute, nor will it print anything. Regardless of whether it’s a function expression or statement, if you don’t call the function, as in the example above, it won’t execute.
funcName ()
Just like this, by adding parentheses at the end, the function will be called.
Function funcName () {
Console.log (“Hello i am from the function” ) ;
}
funcName () ;
> Hello i am from function
“Now function expression”
Var funcName = function() {
console.log(“ i am from the function” )
> i am from the function
The output of the above two codes will be the same. Here, the function is simply displaying the console output inside it. It doesn’t seem like it’s doing much. What we want is to create a function that will take two numbers and return their sum
Function aFunc (parameters){
// do something with the parameters
// return the result
}
aFunc(arguments)
Here comes the discussion of parameters and arguments. We use these to pass data into functions. Now, a function can take as many parameters as we want. It can take not just two, but even a hundred if we wish. However, when we call the function, we must provide exactly the same number of arguments as the number of parameters the function expects. Otherwise, the function won’t give the correct result. Did you encounter any problems with parameters and arguments? The example below will make it clear. When declaring a function, we pass parameters within the first bracket, and when calling the function, we pass arguments
Function sumMachine (a , b ) {
Var sum = a + b ;
Return sum
console.log(sumMachine(2 , 2 ) ) ;
console.log(sumMachine( 4 , 4 ) ;
Here, I have used a function statement, but you can choose to use an expression if you want. We’ll discuss statements and expressions separately later. For now, just remember which one is a statement and which one is an expression.
Here, you should consider using a return statement. We have logged some output to the console using the function above, but it doesn’t produce any value because that function doesn’t return anything. If you want your function to output some value, you need to use a return statement. You can return data of any variable or data type from your function. And inside the function, there will be just one return statement. If you have two return statements, the function will use the first one to return and won’t execute the subsequent one.
Here, a function named sumMachine has been defined with two parameters, a and b. Inside the function, the sum of these two parameters is stored in a variable named sum. Then, we have returned the SUM Now, it’s not specified where to print sum. We have called the function and instructed it to print, “return – 4 & 8.
Now you can pass any variable, string, boolean, array, or object as an argument
Function myName (name , age ) {
console.log(“my name is “ name + “ and i am “ + age + “ years old” )
}
myName(“zonayed ahmed” , 21 ) ;
Output >>> my name is : zonayed ahmed and i am 21 years old
Since functions are considered first-class citizens in JavaScript, you can pass one function as an argument to another function if you wish. Take a look at the following code to see how it’s done properly.
Function callMyName (name , callback ) {
Var myAge = 20;
Callback (myAge ) ;;
console.log(“is it interesting? Yes it is “ + name );
}
Function helloAge (age) {
console.log(“i am pass the argument and my age is : “ + age );
}
callMyName (“zonayed” , hello )
Output >>> i am pass the argument and my age is 20
Is is interesting? yes it is zonayed
function declaration
When we create a function, we actually write the function definition.
Function aSimpleFunction () {
console.log(“asimple function” }
This is how we defined the function. This is the function definition. Then, if we want to call the function, we use parentheses (), which is called function invocation.
aSimpleFunction()
Post Comment