javaScript statement and expression
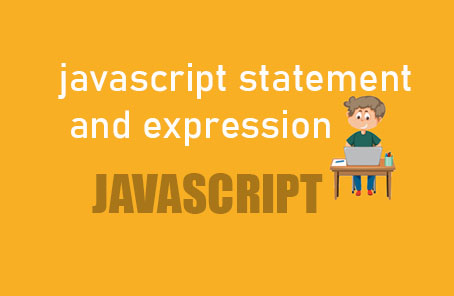
I am using these two words, ‘statement’ and ‘expression,’ in many places. In our code, sometimes we produce values, like 10 + 10 equals 20. Other times, we have lines of code that only access something, like conditional statements. These do not produce any values; they just take specific actions. Based on these, we differentiate between expressions and statements. Simply put, expressions produce values, while statements only take actions.
Expression: Below is an example where 10 is added to 10. Here, a value is produced, so this is an expression.
>>> 10 + 10
Here all are expression
2 + 2
3 + 7
1 + 2 + 3 * ( 8 ** 9 ) – Math.sqrt(4 , 0 )
math.min(2, 22)
Math.max ( 3 , 94 )
Null
True
False
2
4.0
But when these values are assigned somewhere else, then it will be considered as a statement, like the entire following is a statement:
Var assignValue = 10 + 10 ;
Statement: On the other hand, a statement will just take action, like loop conditions and many other things.
if(10 < 20 ) {
console.log(“yes it is true” )
} else {
console.log(“no it is false”);
}
Above, just one action is being taken. Based on the expressions inside, some code has been executed several times, following the same principle, others.
for(expressions) {
// some code
}
Do {
// some code here
} while(expressions) ;
While (expression) ;
while(expression){
// some code here
}
Again, notice that statements can span multiple lines, while expressions often occur on a single line. So, while it can be challenging to distinguish between expressions and statements, there are some differences to consider.
Different kinds of functions: We know that functions can also be stored in variables. Now, if we store a function in a variable, what would it be? An expression or a statement? Yes, according to our discussion, it would indeed be an expression.
In a function expression, when a function is stored in a variable, it becomes a function expression
Var someFunc = function (parameter){
// some code
}
When a function is taken in a normal way, it becomes a function statement
Function aFun(params) {
// code }
Post Comment