DRY principle in javaScript
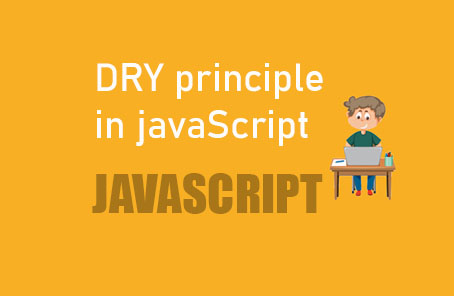
The ‘DRY’ principle, or ‘Don’t Repeat Yourself’, aims to shorten and beautify the process of not doing the same task repeatedly. In programming, situations may arise where we need to write code repeatedly to solve such problems. In such cases, instead of writing the same code repeatedly, we can use certain techniques to make one code do all the work. Additionally, the more lines of code there are, the more difficult it becomes to maintain. Finding problems can also take a lot of time. Therefore, there is no alternative to keeping the code short and beautiful to adhere to the DRY principle.
Let’s start from the very basics. Suppose we want to read a piece of text. Instead of writing it repeatedly, let’s declare a function and use it repeatedly
Function printAText () {
console.log(“this post is written by robin”)
}
So, now wherever we want to print this text, we can simply call the function.
// Some code
printAText() ;
// Some more code
printAText() ;
This way, you can perform tasks without repeatedly writing the same text by using functions. Although this example may not be very effective, it’s good for understanding the basics. Now let’s move on to another example. Suppose we want to find the sum of two numbers. Here, instead of repeatedly adding two numbers, we can use a function that takes the numbers as arguments and returns the sum.
Function addTwoNum ( x , y ) {
Var sum = x + y ;
Console.log (“sum of “ + x + “and” + y + “is : “ + sum ) ;
}
This function will take two arguments and will store their sum. It will then print the details with console.log at the end. Now, every time you call this function with two number arguments, you will be able to see that console log as many times as you call it. You don’t need to keep adding repeatedly.
addTwoNum (5, 10);
addTwoNum (3, 20);
addTwoNum (7, 50);
addTwoNum (8, 80);
So, this way we can maintain the dry principle without repeatedly writing code to print each number or text every time. Now, I’ve introduced the concept of dry principle using only functions. It doesn’t mean that code can only be dried through functions; there are many other ways to dry code. From now on, whenever someone mentions drying code, or you see this term anywhere, I hope you understand what it means. And if you feel like you’re writing the same code repeatedly, then you should try to apply the dry principle there. Many people use the term ‘wet’ as the opposite of dry. It means doing the opposite of dry.
Post Comment