What is Scope in javaScript ?
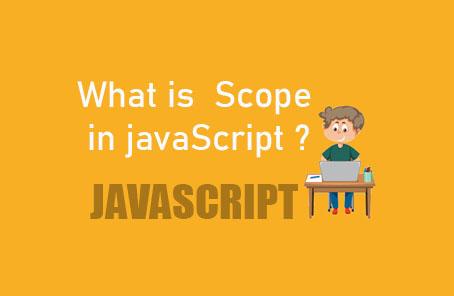
Scoping is another important topic in JavaScript. You need to know where you can access or use your declared variables/functions. If you want to make a variable or function private or accessible from everywhere, you need to know how and where to declare it, which will be discussed within scoping.
There are mainly two types of scoping in JavaScript:
1. Local Scope
2. Global Scope
The main points of discussion in scoping are:
– Where you can access your variables or functions
– Each new function creates a scope
### Lexical Scoping: Parent-Child Relationship
– A variable declared in one function cannot be accessed in another completely separate function.
### 1. Local Scope:
Generally, variables declared inside a function are local variables. These types of variables have local scope, meaning they can only be accessed within the function they were declared in and not outside or in any other function.
“`javascript
function localScope() {
var local = ‘I am local to my own function’;
console.log(local);
}
localScope(); // This will show the correct output
“`
Output:
“`
I am local to my own function
“`
But if we try to access this `local` variable outside the `localScope()` function or in any other function, it will not be accessible and will throw an error.
“`javascript
function localScope() {
var local = ‘I am local to my own function’;
console.log(local);
}
localScope();
console.log(local); // This will throw an error
“`
Error:
“`
Uncaught ReferenceError: local is not defined
“`
### 2. Global Scope:
If a variable is not declared inside any function, then it is a global scope variable, meaning it is accessible from anywhere, including inside functions.
“`javascript
var globalVar = ‘I am a Global Variable’;
function globalScope() {
console.log(‘Inside a Function: ‘ + globalVar);
}
globalScope();
console.log(‘Outside: ‘ + globalVar);
“`
Output:
“`
Inside a Function: I am a Global Variable
Outside: I am a Global Variable
“`
The idea of scoping applies not only to variables but also to functions and objects. If you declare a function in the global scope, it can be accessed from anywhere.
“`javascript
function globalFunc() {
console.log(‘Global Function’);
}
globalFunc(); // This will work correctly
“`
If you declare a function inside another function, the inner function gets the local scope of the outer function and can only be called inside that function.
“`javascript
function anoGlobalFunc() {
console.log(‘Global Function’);
function localFunc() {
console.log(‘Local Function’);
}
localFunc();
}
anoGlobalFunc();
“`
Output:
“`
Global Function
Local Function
“`
But if you try to call this `localFunc()` outside the `anoGlobalFunc()`, it will throw an error.
“`javascript
localFunc(); // This will throw an error
“`
Error:
“`
Uncaught ReferenceError: localFunc is not defined
“`
The same applies to objects. If an object is declared inside a function, it gets the local scope of that function and cannot be accessed outside of it.
“`javascript
function objectFunc() {
var localObj = {
name: ‘Zonayed Ahmed’,
age: 21
};
console.log(localObj);
}
objectFunc();
“`
Output:
“`
{name: “Zonayed Ahmed”, age: 21}
“`
But if you try to access this `localObj` outside the function, it will throw an error.
“`javascript
console.log(localObj); // This will throw an error
“`
Error:
“`
Uncaught ReferenceError: localObj is not defined
“`
### Automatically Global Variables:
In JavaScript, you can declare a variable without using the `var` keyword. In this case, regardless of the scope it is in, it will automatically be considered a global variable.
“`javascript
function autoVar() {
name = ‘Zonayed Ahmed’;
}
autoVar();
console.log(name); // This will work correctly
“`
Output:
“`
Zonayed Ahmed
“`
Remember, you must call the function first before accessing the variable, otherwise, nothing will be executed or run inside the function. This automatically global variable will not work in JavaScript’s ‘strict mode’ and it’s also not a good practice since scoping will not work as you intend. Hence, it’s better to avoid this. ‘Strict mode’ will be discussed later.
### Lexical Scoping:
Lexical scoping creates a parent-child relationship between functions. When you declare a function inside another function, the outer function becomes the parent function, and any function declared inside it becomes its child function.
“`javascript
function parentFunction() {
var a = 6;
function childFunction() {
var b = 4;
console.log(‘Sum: ‘ + (a + b));
}
childFunction();
}
parentFunction();
“`
Output:
“`
Sum: 10
“`
Here, `parentFunction()` is a global function and `childFunction()` is a local function to `parentFunction()`. According to lexical scoping, any local variables, functions, or objects declared inside a parent function can be accessed within the parent function and all its child functions.
Post Comment