What is Closures in javaScript
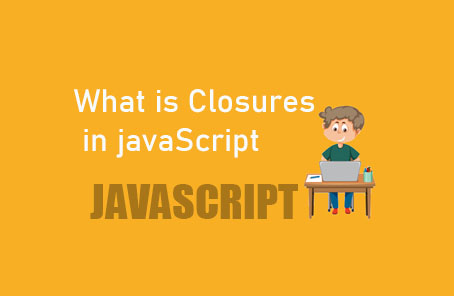
Closures are another topic related to lexical scoping. With closures, we don’t need to do anything separately; rather, it is JavaScript’s default behavior. However, understanding closures is essential for various techniques and to grasp JavaScript’s many surprising behaviors and specialties.
A child function can always access the variables, functions, or objects of its parent function. The more interesting part is that even if we return the parent function, we can still access the parent function’s variables, functions, or objects from the child function. This is because these elements stay with the returned function. And this is what a closure is. It is JavaScript’s default behavior, meaning we don’t need to write separate code for closures.
Let’s understand how this closure works. We know that in JavaScript, you can return another function from within a function. This creates a parent-child relationship. The child function can access everything from the returned function due to the closure.
“`javascript
function closuresDemo() {
var x = 10;
function anotherFunc() {
var y = 20;
console.log(‘Sum: ‘ + (x + y));
}
return anotherFunc;
}
“`
If we call `closuresDemo()`, it will return the inner function `anotherFunc`:
“`javascript
var returnedFunc = closuresDemo();
“`
Here is where the magic happens. We have already returned the parent function `closuresDemo`. However, we still want to access the variable `x` from the inner function `anotherFunc`. Due to lexical scoping, the inner function `anotherFunc` should be able to access the parent function’s variable `x`. But the parent function `closuresDemo` has already returned. To ensure that the inner function can still access `x`, it is packed together with the returned function. This is why we can access `x` even after returning. If we now call that returned function:
“`javascript
returnedFunc();
“`
This essentially calls the child function `anotherFunc`, and we will see the following result:
“`javascript
Sum: 30
“`
Similarly, we can pass arguments and share them from parents to children. This means the child can access the parent’s arguments too.
“`javascript
function aParentFunc(a) {
return function(b) {
console.log(‘Sum: ‘ + (a + b));
};
}
“`
If we call this function with arguments:
“`javascript
aParentFunc(6)(4);
“`
Here, the parent’s argument goes inside the parent function’s parentheses when called, and the child’s argument goes inside the child’s parentheses when called:
“`javascript
Sum: 10
“`
You can also call the parent function first and store it in a variable, then call the child function using that variable:
“`javascript
var aParentVar = aParentFunc(6);
var total = aParentVar(4);
“`
This will show the same result as before. Note that the respective arguments are passed in their respective calls:
“`javascript
Sum: 10
“`
Post Comment