Understanding `call()`, `bind()`, and `apply()` Methods in JavaScript
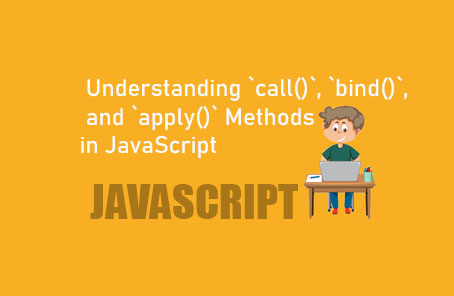
The `this` keyword’s value or context can be determined using four rules, one of which involves explicit rules with `call()`, `bind()`, and `apply()`. All three methods take the `this` context or value as the first argument. Among them, `call()` and `bind()` can accept unlimited arguments, while `apply()` accepts only two arguments: the first to set the value of `this`, and the second, an array, to pass the function’s arguments.
#### `call()` Method
The `call()` method immediately invokes the function with a specified `this` value and individually passed arguments.
##### Example:
Suppose we have an object:
“`javascript
var myCustomObj = {
name: ‘Zonayed Ahmed’,
age: 21,
job: ‘Student’,
anotherObj: {
name: ‘Ahmed Zonayed’,
value: function() {
console.log(‘My name is ‘ + this.name);
}
}
};
“`
Calling the `value()` function directly will output `My name is Ahmed Zonayed`, as `this` refers to `anotherObj`.
“`javascript
myCustomObj.anotherObj.value();
// Output: My name is Ahmed Zonayed
“`
To change the `this` context to `myCustomObj`, use `call()`:
“`javascript
myCustomObj.anotherObj.value.call(myCustomObj);
// Output: My name is Zonayed Ahmed
“`
This demonstrates how `call()` can change the `this` context to another object.
##### Note:
Be careful when using `call()` within an object definition, as it will execute immediately:
“`javascript
var myCustomObj = {
name: ‘Zonayed Ahmed’,
age: 21,
job: ‘Student’,
anotherObj: {
name: ‘Ahmed Zonayed’,
value: function() {
console.log(‘My name is ‘ + this.name);
}.call(myCustomObj)
}
};
“`
This results in:
“`javascript
// Output: My name is Zonayed Ahmed
“`
#### `apply()` Method
The `apply()` method is similar to `call()`, but takes an array of arguments.
##### Example:
Given the same `myCustomObj`:
“`javascript
myCustomObj.anotherObj.value.apply(myCustomObj);
// Output: My name is Zonayed Ahmed
“`
The main difference is how arguments are passed. Consider another example with `karim` and `rahim`:
“`javascript
var karim = {
name: ‘Karim Rahman’,
dob: 1996,
age: function(currentYear, msg) {
console.log(msg + this.name + ‘ is ‘ + (currentYear – this.dob) + ‘ years old!’);
}
};
var rahim = {
name: ‘Rahim Abdu’,
dob: 1986
};
karim.age(2018, ‘Hello World!’);
// Output: Hello World! Karim Rahman is 22 years old!
“`
Using `apply()` with `rahim`:
“`javascript
karim.age.apply(rahim, [2018, ‘Hello World!’]);
// Output: Hello World! Rahim Abdu is 32 years old!
“`
#### `bind()` Method
The `bind()` method is unique in that it doesn’t immediately invoke the function. Instead, it returns a new function with the `this` context set to the provided value.
##### Example:
Using the previous `myCustomObj` example:
“`javascript
var boundFunction = myCustomObj.anotherObj.value.bind(myCustomObj);
boundFunction();
// Output: My name is Zonayed Ahmed
“`
This allows for greater flexibility, as you can store the function and invoke it later.
##### More Advanced Usage:
You can partially apply arguments using `bind()`. For instance, consider `karim` and `rahim`:
“`javascript
var karim = {
name: ‘Karim Rahman’,
dob: 1996,
age: function(currentYear, msg) {
console.log(msg + this.name + ‘ is ‘ + (currentYear – this.dob) + ‘ years old!’);
}
};
var rahim = {
name: ‘Rahim Abdu’,
dob: 1986
};
var rahimAge = karim.age.bind(rahim, 2018);
rahimAge(‘Hello World!’);
// Output: Hello World! Rahim Abdu is 32 years old!
“`
You can also create a reusable function:
“`javascript
var rahimAgeCalculate = karim.age.bind(rahim);
rahimAgeCalculate(2018, ‘Hello Dolly!’);
// Output: Hello Dolly! Rahim Abdu is 32 years old!
“`
In summary, while `call()` and `apply()` invoke functions immediately with a specified `this` context and arguments, `bind()` returns a new function with a fixed `this` context, allowing for more flexible usage and partial application of arguments.
Post Comment