What is Function Constructor and ‘new’ Keyword in JavaScript ?
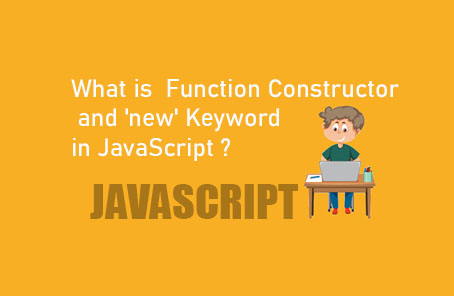
JavaScript is an object-oriented programming language, but those coming from other languages might miss one thing here: the class concept. Despite JavaScript being object-oriented, it operates differently compared to languages like C++ or Java, which are class-based. JavaScript is primarily a prototype-based language. In other languages, everything is encapsulated within classes, but in JavaScript, an object links to another object through a prototype. We previously discussed the prototype chain where objects are linked until the chain ends with `null`, which has no prototype and marks the end of the chain.
JavaScript has built-in constructor functions that create objects and maintain this prototype chain. Some of these built-in constructor functions include:
1. `Array()`
2. `String()`
3. `Number()`
4. `Boolean()`
5. `Date()`
and more…
Using these built-in constructors, we can create arrays, strings, and other objects. These constructors also come with properties and methods that can be used with the created objects. However, we can also create our own constructor functions with desired properties and methods.
Consider we have several objects with similar structures:
“`javascript
var samir = {
name: ‘Samir Hossain’,
age: 22,
job: ‘Student’
};
var kinan = {
name: ‘Kinan Hossain’,
age: 29,
job: ‘Businessman’
};
var jamil = {
name: ‘Jamil Hossain’,
age: 18,
job: ‘Driver’
};
var zawad = {
name: ‘Zawad Ahmed’,
age: 1,
job: ‘Child’
};
var zonayed = {
name: ‘Zonayed Ahmed’,
age: 21,
job: ‘Developer’
};
“`
We see the same pattern repeated for each object. Instead of writing them separately, we can use a constructor function to create a blueprint and then use it to create new objects with the desired values.
Let’s create a constructor function as a blueprint:
“`javascript
var Person = function(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
};
“`
Notice that `Person` starts with a capital P, following the convention for naming constructor functions. The `this` keyword refers to the new object being created when the constructor is invoked with the `new` keyword.
Creating a new object using this constructor:
“`javascript
var samir = new Person(‘Samir Hossain’, 22, ‘Student’);
“`
Here, the `new` keyword sets the context for `this`, assigning it to the newly created object. Therefore, `this` refers to `samir`, and we can access its properties:
“`javascript
console.log(samir.name);
console.log(samir.age);
console.log(samir.job);
“`
Output:
“`
Samir Hossain
22
Student
“`
We can create as many objects as needed using the same constructor:
“`javascript
var kinan = new Person(‘Kinan Hossain’, 29, ‘Businessman’);
var jamil = new Person(‘Jamil Hossain’, 18, ‘Driver’);
var zawad = new Person(‘Zawad Ahmed’, 1, ‘Child’);
var zonayed = new Person(‘Zonayed Ahmed’, 21, ‘Developer’);
“`
This makes the object creation process clean and organized. Additionally, we can add methods to the constructor function:
“`javascript
var PersonWithMethod = function(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
this.dateOfBirth = function() {
console.log(this.name + ‘ is born in ‘ + (2024 – this.age));
};
};
var samirWithMethod = new PersonWithMethod(‘Samir Hossain’, 22, ‘Student’);
samirWithMethod.dateOfBirth(); // Output: Samir Hossain is born in 2002
“`
When logging the object, you’ll see the method included with the properties:
“`javascript
console.log(samirWithMethod);
“`
Output:
“`
PersonWithMethod { name: “Samir Hossain”, age: 22, job: “Student”, dateOfBirth: f }
“`
Creating another object:
“`javascript
var anotherObject = new PersonWithMethod(‘Zawad Ahmed’, 3, ‘Child’);
console.log(anotherObject);
“`
Output:
“`
PersonWithMethod { name: “Zawad Ahmed”, age: 3, job: “Child”, dateOfBirth: f }
“`
However, this approach copies the method for every object, which is inefficient. Instead, we can link the method via the prototype chain, which we’ll discuss next.
Post Comment