What is Inheritance in Object-Oriented Programming (OOP):in javascript
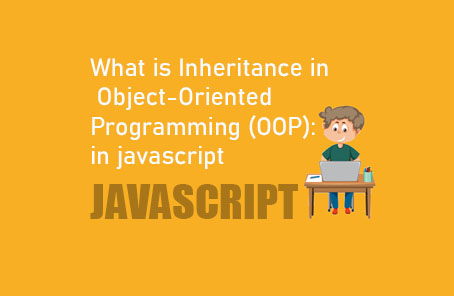
Inheritance is a very important and useful concept in object-oriented programming. With its help, features of one object can be easily taken into another object. Though it might not be clear here, you will understand it properly with a real example.
Suppose, we have a constructor function named `Person`. Now, what might a person have? A name, age, job, etc. So, if we create a blueprint for this, it would look like this:
“`javascript
var Person = function (name, age, job) {
this.name = name;
this.age = age;
this.job = job;
};
“`
Now we can create objects for different persons using this blueprint. But let’s say we want another blueprint for `Teacher`. What might a teacher have? Assume that a teacher also has a name, age, job, and additionally a subject they teach. Now, if we want to create a blueprint for the teacher:
“`javascript
var Teacher = function (name, age, job, subject) {
this.name = name;
this.age = age;
this.job = job;
this.subject = subject;
};
“`
But reusing the same properties like this doesn’t look very clean. Because, in reality, a teacher is also a person who just has a few additional properties compared to a regular person. We want to do something here so that we can bring the features of `Person` into `Teacher` and define the additional properties of the teacher. This is essentially what inheritance means. Inheritance can be of several types. I will discuss it in detail below.
### Prototype-Based Inheritance
Let’s assume, in the previous section, we created a constructor function like this:
“`javascript
var PersonOnly = function (name, age, job) {
this.name = name;
this.age = age;
this.job = job;
};
“`
Assume we want to keep a method to calculate the date of birth, but as we saw in the previous topic, keeping the method directly in the constructor means it gets copied into each created object repeatedly. We can solve this problem by using the prototype to define this new method, which will not be copied repeatedly into newly created objects but can still be accessed by those objects due to the prototype chain:
“`javascript
PersonOnly.prototype.dateOfBirth = function () {
console.log(this.name + ‘ born in ‘ + (2018 – this.age));
};
“`
Now, write this in the console:
“`javascript
console.log(PersonOnly.prototype);
“`
You will see our newly created method there:
“`javascript
> console.log(PersonOnly.prototype);
▾ {dateOfBirth: f, constructor: f}
dateOfBirth: f ()
constructor: f (name, age, job)
__proto__: Object
“`
Now, we know about the prototype chain from the previous section. Due to the prototype chain, this method can now be accessed by objects created from `PersonOnly`. Suppose we create an object from this constructor function:
“`javascript
var zawad = new PersonOnly(‘Zawad Ahmed’, 1, ‘Child’);
“`
Now, if we want to access our created method:
“`javascript
zawad.dateOfBirth();
“`
The output will be:
“`javascript
Zawad Ahmed born in 2017
“`
But here’s the interesting part. If we look at the object `zawad` created by `PersonOnly` in the console:
“`javascript
console.log(zawad);
“`
You will see an interesting change:
The method `dateOfBirth` is not there, but we could still call it using `zawad.dateOfBirth`. The reason is, if we open `__proto__` in the console, we will find our object there:
“`javascript
> console.log(zawad);
PersonOnly {name: “Zawad Ahmed”, age: 1, job: “Child”}
__proto__: Object
“`
Due to the prototype chain, we can access this method, and it is not being copied with every new object. We will see the same result if we create another object:
“`javascript
var anotherObject = new PersonOnly(‘Another Person’, 34, ‘Jobless’);
console.log(anotherObject);
“`
The output will be:
“`javascript
> console.log(anotherObject);
PersonOnly {name: “Another Person”, age: 34, job: “Jobless”}
__proto__: Object
dateOfBirth: f ()
constructor: f (name, age, job)
__proto__: Object
“`
The method is essentially connected to the prototype of the constructor function (which we did when creating the method: `PersonOnly.prototype.dateOfBirth`), and due to the prototype chain, we can easily access it with our created objects.
In the same way, we can add variables to the constructor function’s prototype. For example, we add a new variable to `PersonOnly`:
“`javascript
PersonOnly.prototype.address = ‘Bangladesh’;
“`
Now, we can access this from the `zawad` object as well:
“`javascript
console.log(zawad.address);
“`
The output will be:
“`javascript
Bangladesh
“`
Magic! This is the magic of prototype inheritance. Now let’s move on to something bigger.
### Constructor Inheritance
This is what we discussed at the beginning. There is only one difference between a person and a teacher. Now, do we need to create two separate constructor functions for this? Definitely not; this is where inheritance comes into play.
Suppose we have a constructor function named `Person`:
“`javascript
var Person = function (name, age, job) {
this.name = name;
this.age = age;
this.job = job;
};
“`
Now, we need a constructor function for teachers as well, which will have these properties along with an additional `subject` property. The constructor function for this could be:
“`javascript
var Teacher = function (name, age, job, subject) {
this.name = name;
this.age = age;
this.job = job;
this.subject = subject;
};
“`
But because we have inheritance, there is no need to redefine everything in `Teacher`. We can easily bring `name`, `age`, and `job` from `Person` like this:
“`javascript
var Teacher = function (name, age, job, subject) {
Person.call(this, name, age, job);
this.subject = subject;
};
“`
Does something new seem to be happening? Yes, it’s a bit complex to understand. Here, we have called `Person` inside and used the `call` method to set the value of `this`. Now, `this` here means `this`! Why? Yes, try to recall. When we create an object with this, we will use the `new` keyword. And because of that, a new context for `this` will be created, which will refer to the new object. This means that the `this` defined here actually refers to the object we will create in the future. Now, the rest of the parts are just like before:
“`javascript
var kamaljeet = new Teacher(‘Kamaljeet Saini’, 53, ‘Teacher’, ‘CSE’);
“`
That’s it, we can create a new object like this. It will work correctly:
“`javascript
console.log(kamaljeet.name);
console.log(kamaljeet.age);
console.log(kamaljeet.job);
console.log(kamaljeet.subject);
“`
The output will be:
“`javascript
Kamaljeet Saini
53
Teacher
CSE
“`
That’s it! This is how we inherited the features of `Person` into `Teacher`.
Post Comment