Explanation of Immediately Invoked Function Expressions (IIFE)
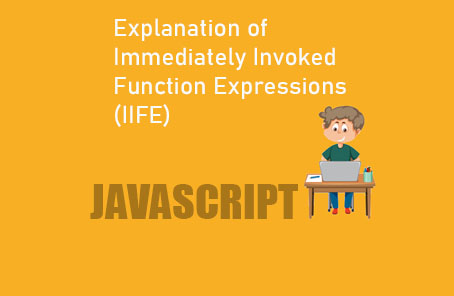
In ES5, we learned about Immediately Invoked Function Expressions (IIFE), their usage, and how they work in detail. IIFEs are particularly useful when you want to maintain privacy or prevent data inside a function from being exposed outside. For example:
“`javascript
(function demoFunc() {
var msg = ‘Hello World’;
console.log(msg);
})();
“`
This will directly output:
“`
Hello World
“`
If we try to call this function again from outside, it will show an error:
“`javascript
demoFunc();
“`
“`
Uncaught ReferenceError: demoFunc is not defined at <anonymous>:1:1
“`
Similarly, if we try to access the variable `msg`, it will also be impossible:
“`javascript
console.log(msg);
“`
“`
Uncaught ReferenceError: msg is not defined at <anonymous>:1:13
“`
Using IIFE, you can create private functions and variables this way. However, with ES6’s `let` or `const` block-level scoping, this becomes much simpler. If you want to create private functions or variables, just declare them with `let` or `const` (not `var` due to differences in scoping) and enclose them in braces `{}` to make them private and inaccessible from outside:
“`javascript
{
let a = 5;
let b = 10;
const pi = 3.1416;
console.log(a + b + pi);
}
“`
This will correctly output:
“`
18.1416
“`
But if you try to access these variables from outside the braces:
“`javascript
console.log(a + b + pi);
“`
“`
Uncaught ReferenceError: a is not defined at <anonymous>:6:13
“`
The same principle applies to functions. If we declare a function expression with `var`, its scope will be according to `var`’s scope. Even if you enclose it within braces, it can still be accessed from outside:
“`javascript
{
var myName = function() {
var a = ‘Zonayed Ahmed’;
console.log(a);
}
}
myName();
“`
This will correctly output:
“`
Zonayed Ahmed
“`
However, if the same function is declared with `let` or `const`, it will become a private function due to block scoping:
“`javascript
{
const myName6 = function() {
const a = ‘Zonayed Ahmed’;
console.log(a);
}
}
myName6();
“`
This will show an error:
“`
Uncaught ReferenceError: myName6 is not defined at <anonymous>:5:1
“`
This way, you can easily achieve the functionality of IIFE in ES6.
Post Comment