What is Template Literals in javascript ?
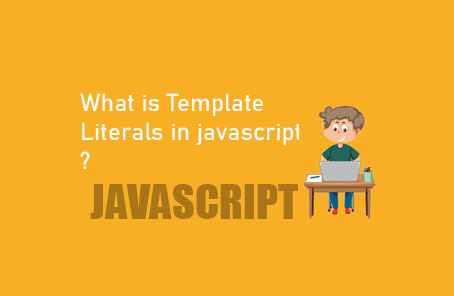
“`javascript
// Declaring variables
var name = ‘Zonayed Ahmed’,
age = 21,
work = ‘Student’;
// Using traditional string concatenation
console.log(‘My name is ‘ + name + ‘ and I\’m ‘ + age + ‘ years old! Currently I\’m a ‘ + work);
// Using template literals
const name6 = ‘Zonayed Ahmed’,
age6 = 21,
work6 = ‘Student’;
console.log(`My name is ${name6} and I’m ${age6} years old! Currently I’m a ${work6}`);
“`
In JavaScript, if we want to print a string along with some variables, we usually have to concatenate those variables using the `+` operator. This process can be quite tedious, especially for long strings, where it’s easy to get lost. For instance, let’s say I want to print some information from a few variables:
“`javascript
var name = ‘Zonayed Ahmed’,
age = 21,
work = ‘Student’;
console.log(‘My name is ‘ + name + ‘ and I\’m ‘ + age + ‘ years old! Currently I\’m a ‘ + work);
“`
Executing this will output:
“`
My name is Zonayed Ahmed and I’m 21 years old! Currently I’m a Student
“`
Here, we have to concatenate the variables with the string using the `+` operator. This works, but repeatedly binding strings with `+` to access variables is cumbersome. For long strings, it’s easy to miss a `+` or two. Also, notice that to write `I’m`, we had to use the escape character `\`. Overall, it becomes a mess.
To address this issue, ES6 introduced template literals. The main purpose of template literals is to reduce the complexity of string concatenation. With template literals, we use the backtick (located above the tab key) to enclose the entire string, and for any variables or simple calculations, we use `${}` inside the string. If we print the same thing using template literals:
“`javascript
const name6 = ‘Zonayed Ahmed’,
age6 = 21,
work6 = ‘Student’;
console.log(`My name is ${name6} and I’m ${age6} years old! Currently I’m a ${work6}`);
“`
The output will be:
“`
My name is Zonayed Ahmed and I’m 21 years old! Currently I’m a Student
“`
Here, I declared the variables using `const`, but you can use `var` or `let` if you prefer. Since I’m writing in ES6, I used `const`. This code is definitely less hassle and looks much cleaner than the previous one. Additionally, you can use any character without escaping. Smart!
Some more examples with template literals:
“`javascript
function calculateAge(dob) {
return `I’m ${2021 – dob} years old!`;
}
console.log(calculateAge(1996));
“`
Another thing about template literals is that they remember everything you write inside the backticks, including new lines and spaces. You will get the output exactly as you write it:
“`javascript
console.log(`Hello World!
How are you?
I am Fine…
And You?`);
“`
The output will be exactly like this:
“`
Hello World!
How are you?
I am Fine…
And You?
“`
### Tagged Templates
Another use case of template literals is tagged templates. It’s like a function call, but here, the arguments are taken using template literals. Here’s an example to clarify:
A regular function call:
“`javascript
aFunc();
“`
And a tagged function:
“`javascript
aFunc`I’m ${name}. I’m ${age} years old.`;
“`
Here, just the function name is placed before the template literal. Essentially, this is a syntactic sugar. If we write the above tagged function as a normal function call:
“`javascript
aFunc([“I’m “, ” years old.”], name, age);
“`
The contents inside the template literal are passed as arguments, which we can then receive in our function and manipulate as we like. If we define our `aFunc` like this:
“`javascript
const aFunc = (strings, name, age) => {
console.log(‘Strings:’, strings);
console.log(`Name: ${name}`);
console.log(`Age: ${age}`);
};
“`
Then, calling it with the template tag:
“`javascript
const name = ‘Zonayed Ahmed’;
const age = 23;
aFunc`I’m ${name}. I’m ${age} years old.`;
“`
You’ll get an output like this:
“`
Strings: [“I’m “, “. I’m “, ” years old.”]
Name: Zonayed Ahmed
Age: 23
“`
As you can see, the `strings` array contains the parts of the string, and we can rearrange and manipulate them as we like using the function.
Post Comment