Advance javascript String Methods
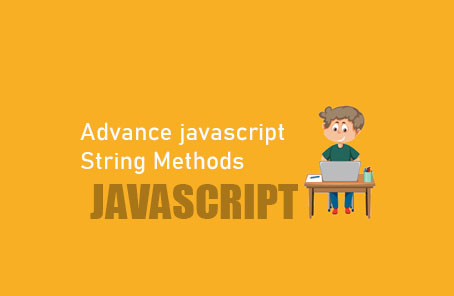
“`javascript
// startsWith Method
const start = ‘My name is Khan’;
console.log(start.startsWith(‘My’)); // true
// endsWith Method
const end = ‘I love programming’;
console.log(end.endsWith(‘My’)); // false
// includes Method
const inc = ‘I love programming with JavaScript’;
console.log(inc.includes(‘with’)); // true
// repeat Method
const rpt = ‘Hello World ‘;
console.log(rpt.repeat(5)); // “Hello World Hello World Hello World Hello World Hello World “
// Additional example with repeat
console.log(`I always want to say ${‘Alhamdulillah ‘.repeat(5)}!`);
// I always want to say Alhamdulillah Alhamdulillah Alhamdulillah Alhamdulillah Alhamdulillah !
“`
String Methods
With ES6, some new string methods have been introduced. Here, I will discuss some very useful methods among them.
– To check if a string starts with a specific character/string, use the `startsWith` method.
– To check if a string ends with a specific character/string, use the `endsWith` method.
– To check if a string contains a specific character/string, use the `includes` method.
– To repeat a string a specific number of times, use the `repeat` method.
#### startsWith Method
This method is used to check if your desired string starts with a specific character or set of characters. It always returns a Boolean value, either true or false:
“`javascript
const start = ‘My name is Khan’;
console.log(start.startsWith(‘My’)); // true
“`
#### endsWith Method
This method is used to check if your desired string ends with a specific character or set of characters. It also always returns a Boolean value, either true or false:
“`javascript
const end = ‘I love programming’;
console.log(end.endsWith(‘My’)); // false
“`
#### includes Method
This method is used to check if your desired string contains a specific character or set of characters. It also always returns a Boolean value, either true or false:
“`javascript
const inc = ‘I love programming with JavaScript’;
console.log(inc.includes(‘with’)); // true
“`
#### repeat Method
This method is used to repeat a string a specific number of times:
“`javascript
const rpt = ‘Hello World ‘;
console.log(rpt.repeat(5)); // “Hello World Hello World Hello World Hello World Hello World “
“`
#### Additional Example
“`javascript
console.log(`I always want to say ${‘Alhamdulillah ‘.repeat(5)}!`);
// I always want to say Alhamdulillah Alhamdulillah Alhamdulillah Alhamdulillah Alhamdulillah !
“`
Remember, these are string methods and will only work with strings, not with numbers or anything else. Although I have used `const` in the examples, you can also use `var` or `let`. I just wanted to keep everything in ES6 as much as possible since I am writing the code in ES6.
Post Comment