What is Arrow Functions in javascript ?
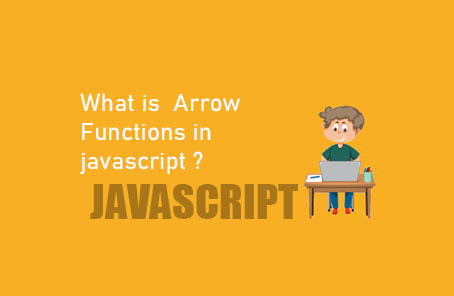
A new addition to JavaScript’s ES6 is the arrow function. Many also refer to it as the fat arrow function. It’s not actually something new, but rather syntactically pleasing and clean. In the programming world, there’s a term called “syntactic sugar.” It means syntax that looks nice and feels clean. Although the functionality of arrow functions is quite similar to ES5 functions, there are some differences.
In ES5, we write a function expression like this:
“`javascript
var aFunc = function() {
console.log(‘A Demo ES5 Function Expression’);
}
“`
Now, if we call this function:
“`javascript
aFunc();
“`
Output:
“`
A Demo ES5 Function Expression
“`
If we write the same function using an arrow function in ES6:
“`javascript
const aFunc6 = () => console.log(‘A Demo ES6 Arrow Function’);
“`
Now, if we run this function:
“`javascript
aFunc6();
“`
Output:
“`
A Demo ES6 Arrow Function
“`
Here are the differences between them. In the ES6 arrow function, we don’t need the `function` keyword. Instead, we use a new syntax `=>`. That’s why it’s called an arrow function. Also, the code looks much cleaner with arrow functions. Where we had to write several lines or use `{}` brackets in ES5 functions, we don’t need them in arrow functions.
### Automatic Return
Arrow functions are quite smart. Here’s an example demonstrating their smartness. Suppose we have an array like this:
“`javascript
const dob = [1996, 1986, 2017, 1989];
“`
Now, I want to use `map()` to calculate the current age for each year in the array. That means I want to create another array with the age by comparing the years in the array with the current year (2018 at the time of writing). First, in ES5:
“`javascript
const currentAge5 = dob.map(function(oneDob) {
return 2018 – oneDob;
});
“`
Now, `currentAge5` will have an array of everyone’s current age.
“`javascript
currentAge5; // Output: [22, 32, 1, 29]
“`
If we write the same code using an arrow function in ES6:
“`javascript
const currentAge6 = dob.map(oneDob => 2018 – oneDob);
“`
Now, if we print this:
“`javascript
currentAge6; // Output: [22, 32, 1, 29]
“`
Notice how the code is much shorter and cleaner with arrow functions. Another thing to note is that we didn’t have to use `return` in the arrow function. Because if there is a one-liner expression and there’s something to return, the arrow function automatically returns it.
### Multiple Arguments
In the above example, we had only one argument. Now, we know `map()` takes three arguments. If there are multiple arguments, they must be inside `()` brackets in arrow functions. Even if there’s only one, you can put it in brackets, but the less code, the better. In the previous example, if there were two arguments:
“`javascript
const currentAge62 = dob.map((oneDob, index) => `${index}: ${2018 – oneDob}`);
“`
Now, if we run this:
“`javascript
currentAge62; // Output: [“0: 22”, “1: 32”, “2: 1”, “3: 29”]
“`
### Multiple Lines
What if there are multiple lines or statements? In that case, it won’t automatically return, and we need to use the `return` keyword just like in ES5:
“`javascript
const currentAge622 = dob.map(oneDob => {
const age = 2018 – oneDob;
return age;
});
“`
Now, if we run this:
“`javascript
currentAge622; // Output: [22, 32, 1, 29]
“`
Notice another thing here. In the case of arrow functions, we have to use function expressions. Even in ES5 function expressions, due to hoisting, we can’t call a function before defining it. In ES5 function statements, it was possible due to hoisting. But with ES6 arrow functions, since they are function expressions, you can’t call a function before defining it:
“`javascript
myNameBefore6();
const myNameBefore6 = () => console.log(‘My name is Zonayed’);
“`
This will give an error:
“`javascript
myNameBefore6(); // Uncaught ReferenceError: myNameBefore6 is not defined
“`
Here, I used `const` for variable declaration, but you can use `let` or `var`. The result won’t be different.
Post Comment