Lexical ‘this’ Keyword in javaScript
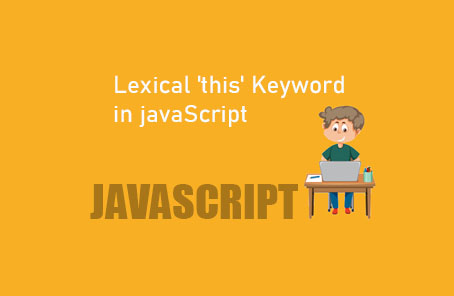
We explain the code billow
“`javascript
// Using ES5 function
const lex5 = {
aFunc: function () {
console.log(this);
return function () {
console.log(this);
};
}
};
lex5.aFunc()();
// Using ES6 arrow function
const lex6 = {
aFunc: () => {
console.log(this);
return () => {
console.log(this);
};
}
};
lex6.aFunc()();
// Mixing ES5 function and ES6 arrow function
const lex56 = {
aFunc: function () {
console.log(this);
return () => {
console.log(this);
};
}
};
lex56.aFunc()();
// Using IIFE with arrow function
(() => {
console.log(‘My name is Zonayed Ahmed’);
})();
“`
Lexical ‘this’ Keyword
So far, what we have discussed are mostly syntactic sugars. The biggest difference between arrow functions and normal functions lies in the `this` keyword. We know that in ES5, the value of `this` in a function depends a lot on how the function is called. Each defined function in ES5 influences the value of `this`. However, the ES6 arrow function does not have its own impact on the `this` keyword. Instead, it takes the `this` value from its surrounding context.
Let’s take this example for better understanding. First, using ES5 functions:
“`javascript
const lex5 = {
aFunc: function () {
console.log(this);
return function () {
console.log(this);
};
}
};
lex5.aFunc()();
“`
In this code, there are multiple functions within an object. In the first function, `this` correctly refers to the object `lex5`. But the inner function refers to the global object. When you call both functions:
“`javascript
lex5.aFunc()();
// Output:
// {aFunc: f}
// Window {postMessage: f, blur: f, focus: f, close: f, frames: Window, …}
“`
You see that the first one refers to the object `lex5`, but the inner function refers to the global object. This happens because the value of `this` in ES5 depends on how the function is called. But if we use the same code with ES6 arrow functions:
“`javascript
const lex6 = {
aFunc: () => {
console.log(this);
return () => {
console.log(this);
};
}
};
lex6.aFunc()();
// Output:
// Window {postMessage: f, blur: f, focus: f, close: f, frames: Window, …}
// Window {postMessage: f, blur: f, focus: f, close: f, frames: Window, …}
“`
Both times, it refers to the global object. The `this` keyword has no impact inside arrow functions. The surrounding `this` is used inside the arrow function as well. For example, if we mix two functions in this example:
“`javascript
const lex56 = {
aFunc: function () {
console.log(this);
return () => {
console.log(this);
};
}
};
lex56.aFunc()();
// Output:
// {aFunc: f}
// {aFunc: f}
“`
The `this` in the outer ES5 function remains the same inside the arrow function. This is known as the lexical `this` keyword in arrow functions. It is very useful. Previously, whenever we created a new function, we had to think about what the value of `this` would be. Now, we don’t need to do that because we know that arrow functions have no impact on `this`.
In ES5, we could explicitly set the value of `this` using the `call`, `bind`, or `apply` methods. But we can’t do that with arrow functions in ES6. Through lexical scoping, it uses the value of `this` from its surrounding context. Although this might seem disadvantageous, it is actually very flexible. You will get used to it as you continue to use it.
We can also use IIFE with arrow functions like this:
“`javascript
(() => {
console.log(‘My name is Zonayed Ahmed’);
})();
“`
Output:
“`javascript
My name is Zonayed Ahmed
“`
Post Comment