What is Destructuring in javascript?
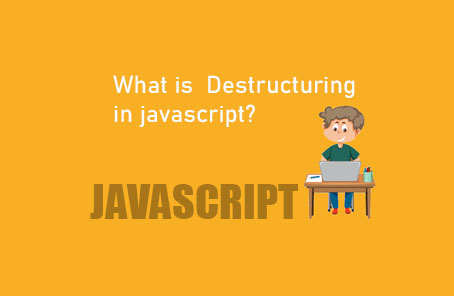
JavaScript ES6 and later versions are primarily designed for smart programming. By using ES6, you will become more efficient, enabling you to do more with less code. Destructuring serves this purpose. It is not a new feature but rather a technique, a smart programming syntactic sugar.
The main task of destructuring is to extract data from a data structure like an array or an object. How is this smart? We will see with examples from both ES5 and ES6.
Let’s say we have the following array:
“`javascript
const zonayed = [‘Zonayed Ahmed’, 21, ‘Student’];
“`
Now, we want to extract these data into separate variables:
“`javascript
var name = zonayed[0];
var age = zonayed[1];
var profession = zonayed[2];
“`
This way, we can extract the data from the array into separate variables in ES5. Here, `name`, `age`, and `profession` are three separate variables holding our desired data.
“`javascript
console.log(name);
console.log(age);
console.log(profession);
// Output:
// Zonayed Ahmed
// 21
// Student
“`
Not bad, but this can be tedious with a lot of data or when done repeatedly. This is where ES6 destructuring comes in. To do the same task in ES6:
“`javascript
const [name6, age6, profession6] = zonayed;
“`
Done! All your work is now done in one line. Here, `name6`, `age6`, and `profession6` are three separate variables that hold the values from the array based on their positions. Accordingly, `name6 = ‘Zonayed Ahmed’`, `age6 = 21`, and `profession6 = ‘Student’`.
“`javascript
console.log(name6);
console.log(age6);
console.log(profession6);
// Output:
// Zonayed Ahmed
// 21
// Student
“`
We can do the same with objects. However, here we have more flexibility. Let’s assume we have the following object:
“`javascript
const zonayedObj = {
nameObj: ‘Zonayed Ahmed’,
ageObj: 21,
professionObj: ‘Student’
};
“`
Since objects have both values and keys, destructuring is quite flexible here. First, let’s see how to extract values in ES5:
“`javascript
var nameObj5 = zonayedObj.nameObj;
var ageObj5 = zonayedObj.ageObj;
var professionObj5 = zonayedObj.professionObj;
“`
Here, the values will be stored in `nameObj5`, `ageObj5`, and `professionObj5` respectively.
“`javascript
console.log(nameObj5);
console.log(ageObj5);
console.log(professionObj5);
// Output:
// Zonayed Ahmed
// 21
// Student
“`
Now, the same task in ES6 can be done in many ways. Since object type data structures have keys, we can easily use the default key names:
“`javascript
const { nameObj, ageObj, professionObj } = zonayedObj;
“`
Now, `nameObj`, `ageObj`, and `professionObj` are independent variables holding their respective values.
“`javascript
console.log(nameObj);
console.log(ageObj);
console.log(professionObj);
// Output:
// Zonayed Ahmed
// 21
// Student
“`
Since the keys inside the object had these names, we could destructure them with the same names. But if we want to destructure with different names, we can do it this way:
“`javascript
const { nameObj: nameObj6, ageObj: ageObj6, professionObj: professionObj6 } = zonayedObj;
“`
Now, `nameObj6`, `ageObj6`, and `professionObj6` are three variables holding their respective values:
“`javascript
console.log(nameObj6);
console.log(ageObj6);
console.log(professionObj6);
// Output:
// Zonayed Ahmed
// 21
// Student
“`
Destructuring objects can go much deeper. Let’s say we have an object within an object:
“`javascript
var comObj = {
anotherObj: {
anotherNewObj: {
title: ‘JavaScript ES6’
}
}
};
“`
To destructure `title` into a variable named `title`:
“`javascript
const { anotherObj: { anotherNewObj: { title } } } = comObj;
“`
Now, `title` is another variable:
“`javascript
console.log(title);
// Output:
// JavaScript ES6
“`
If you want `title` under a different name, you can mix the techniques:
“`javascript
const { anotherObj: { anotherNewObj: { title: newTitle } } } = comObj;
“`
Now, the `title` will be stored in the variable `newTitle`, and you can access it using this variable:
“`javascript
console.log(newTitle);
// Output:
// JavaScript ES6
“`
Post Comment