JavaScript Code of es6 advance array
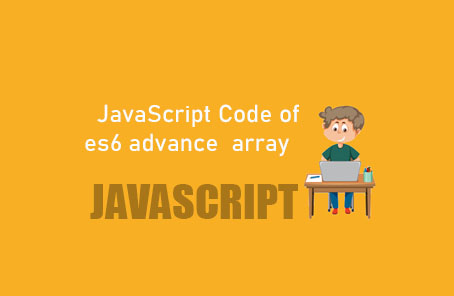
“`javascript
// Converting a NodeList to an array in ES5
const nodeToArr = Array.prototype.slice.call(nodeList);
// Converting a NodeList to an array in ES6
const nodeToArr6 = Array.from(nodeList);
// Looping with break using for loop in ES5
var numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
for (var i = 0; i < numbers.length; i++) {
if (numbers[i] === 5) break;
console.log(‘Number printed: ‘ + numbers[i]);
}
// Looping with break using for…of loop in ES6
for (const number of numbers) {
if (number === 5) break;
console.log(`Number printed: ${number}`);
}
// Finding an element in an array using map in ES5
var numbers = [19, 23, 14, 56, 32, 65, 10, 45];
var findNum = numbers.map(function (theNum) {
return theNum === 65;
});
console.log(findNum);
console.log(findNum.indexOf(true)); // 5
console.log(numbers[findNum.indexOf(true)]); // 65
// Finding an element’s index in an array using findIndex in ES6
numbers.findIndex(number => number === 65); // 5
“`
Explain
Arrays are nothing new in JavaScript. But ES6 introduces some new array methods. As mentioned earlier, ES6 comes with new techniques designed to make our lives easier. To simplify our lives, powerful new methods have been introduced.
When working with the DOM, we often encounter objects that look like arrays. One of the most common such objects is a NodeList. Although a NodeList looks a lot like an array and even has a length property, it is not actually an array. This can cause issues when you try to use array methods on a NodeList. To avoid these issues, you can convert the NodeList to an array.
Suppose we have a NodeList named nodeList. In ES5, you can convert this NodeList to an array using:
“`javascript
const nodeToArr = Array.prototype.slice.call(nodeList);
“`
This converts the NodeList to an array, but the code is somewhat complex and not very readable. In ES6, a new method called Array.from makes this conversion much simpler:
“`javascript
const nodeToArr6 = Array.from(nodeList);
“`
Now, nodeToArr6 is the array version of our NodeList. This code is much easier to understand and less error-prone.
### Looping
To loop through each item in an array and perform operations, we have smart methods like forEach, map, and others. However, in certain situations, these methods may not be useful because we cannot use break or continue statements within them. In such cases, we might need to use a for loop.
Suppose we have the following array:
“`javascript
var numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
“`
If we want to perform an operation up to number 5 and then break, we can use the following ES5 for loop:
“`javascript
for (var i = 0; i < numbers.length; i++) {
if (numbers[i] === 5) break;
console.log(‘Number printed: ‘ + numbers[i]);
}
“`
This requires a lot of work for a simple task. Also, if a semicolon is mistakenly replaced by a comma within the loop, the code will not work. These types of loops can be quite cumbersome. ES6 introduces a simpler solution using the for…of loop:
“`javascript
for (const number of numbers) {
if (number === 5) break;
console.log(`Number printed: ${number}`);
}
“`
Now, if we want to find an element in an array, we had to write a lot of code in ES5. Suppose we want to check if the number 65 exists in the following array:
“`javascript
var numbers = [19, 23, 14, 56, 32, 65, 10, 45];
“`
In ES5, we would do:
“`javascript
var findNum = numbers.map(function (theNum) {
return theNum === 65;
});
console.log(findNum);
console.log(findNum.indexOf(true)); // 5
console.log(numbers[findNum.indexOf(true)]); // 65
“`
This process of finding the index and then the actual element is quite complex. ES6 introduces new methods like filter, some, and every, but for finding the index, the new findIndex method simplifies the process:
“`javascript
numbers.findIndex(number => number === 65); // 5
“`
With the new ES6 techniques, these tasks become much easier to perform.
Post Comment