What is . Rest Parameter in javascript
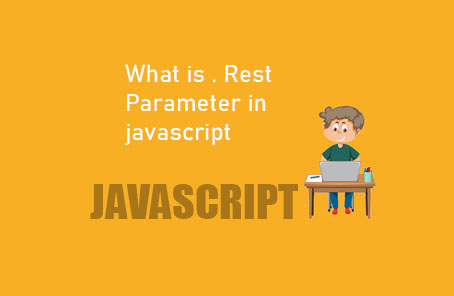
The rest parameter allows you to pass any number of parameters into a function. When we define a function with parameters, if it is unknown how many arguments might be passed, we use the rest parameter or the arguments object from ES5. The arguments object in JavaScript ES5 essentially did the same job, but the rest parameter in ES6 is a bit smarter. The rest parameter uses the same three dots (ellipsis) as the spread operator. Therefore, you need to understand when these dots are used as a rest parameter and when they are used as a spread operator.
Though similar to the previous arguments object, the rest parameter is a bit different. In JavaScript ES6, the new arrow functions do not support the arguments object. So, when you use arrow functions, you must use the rest parameter instead. Additionally, the rest parameter is much more flexible than the arguments object. You will understand better with the example below.
In ES5, when using the arguments object, the arguments passed are stored in an array-like object. You then need to extract each argument one by one from that object:
“`javascript
function arguments5() {
for (var i = 0; i < arguments.length; i++) {
console.log(‘Argument passed: ‘ + arguments[i]);
}
}
arguments5(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Argument passed: Bangladesh
// Argument passed: India
// Argument passed: Sri Lanka
arguments5(‘Cricket’, ‘Football’, ‘Volleyball’, ‘Kabadi’, ‘Kutkut’);
// Output:
// Argument passed: Cricket
// Argument passed: Football
// Argument passed: Volleyball
// Argument passed: Kabadi
// Argument passed: Kutkut
“`
But the same thing won’t work with arrow functions:
“`javascript
const argumentsArr = () => {
for (var i = 0; i < arguments.length; i++) {
console.log(‘Argument passed: ‘ + arguments[i]);
}
};
argumentsArr(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Uncaught ReferenceError: arguments is not defined
“`
Similarly, since the arguments object is not an actual array, you cannot directly use methods like forEach, map, filter, reduce, etc., on it:
“`javascript
function argumentsMeth() {
arguments.map(function (oneArgs) {
console.log(‘Argument Passed: ‘ + oneArgs);
});
}
argumentsMeth(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Uncaught TypeError: arguments.map is not a function
“`
This is because the arguments object does not have these methods. However, you can use a trick to inherit these methods from the Array prototype:
“`javascript
function argumentsMethWork() {
var argsArr = Array.prototype.slice.call(arguments);
argsArr.map(function (oneArgs) {
console.log(‘Argument Passed: ‘ + oneArgs);
});
}
argumentsMethWork(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Argument Passed: Bangladesh
// Argument Passed: India
// Argument Passed: Sri Lanka
“`
Now that we have seen the pros and cons of the arguments object, let’s look at how the rest parameter works. We will redo the same program using the rest parameter:
“`javascript
function arguments6(…anyName) {
for (var i = 0; i < anyName.length; i++) {
console.log(‘Argument passed: ‘ + anyName[i]);
}
}
arguments6(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Argument passed: Bangladesh
// Argument passed: India
// Argument passed: Sri Lanka
arguments6(‘Cricket’, ‘Football’, ‘Volleyball’, ‘Kabadi’, ‘Kutkut’);
// Output:
// Argument passed: Cricket
// Argument passed: Football
// Argument passed: Volleyball
// Argument passed: Kabadi
// Argument passed: Kutkut
“`
The first thing you will notice is the three dots, which are used in the rest parameter. The second thing is that you can name your parameter anything you like. This works perfectly with arrow functions as well:
“`javascript
const argumentsArr6 = (…anyName) => {
for (var i = 0; i < anyName.length; i++) {
console.log(‘Argument passed: ‘ + anyName[i]);
}
};
argumentsArr6(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Argument passed: Bangladesh
// Argument passed: India
// Argument passed: Sri Lanka
argumentsArr6(‘Cricket’, ‘Football’, ‘Volleyball’, ‘Kabadi’, ‘Kutkut’);
// Output:
// Argument passed: Cricket
// Argument passed: Football
// Argument passed: Volleyball
// Argument passed: Kabadi
// Argument passed: Kutkut
“`
Similarly, you can use methods like forEach, map, filter, reduce, etc., directly:
“`javascript
const argumentsMeth6 = (…anyName) => {
anyName.map(oneArgs => console.log(‘Argument Passed: ‘ + oneArgs));
};
argumentsMeth6(‘Bangladesh’, ‘India’, ‘Sri Lanka’);
// Output:
// Argument Passed: Bangladesh
// Argument Passed: India
// Argument Passed: Sri Lanka
“`
This works without any modifications. Moreover, the rest parameter has another major advantage. If you already know some parameters beforehand, you can define them, and then use the rest parameter for the unknown or variable number of parameters:
“`javascript
const restExtra = (name, age, …others) => {
console.log(`My name is ${name} and I am ${age} years old!`);
others.map(other => console.log(`Argument Passed: ${other}`));
};
restExtra(‘Zonayed Ahmed’, 21, ‘JavaScript’, ‘Chrome’, ‘Web Developer’, ‘Front End Engineer’);
// Output:
// My name is Zonayed Ahmed and I am 21 years old!
// Argument Passed: JavaScript
// Argument Passed: Chrome
// Argument Passed: Web Developer
// Argument Passed: Front End Engineer
“`
Post Comment