What is . Default Parameters in javascript ?
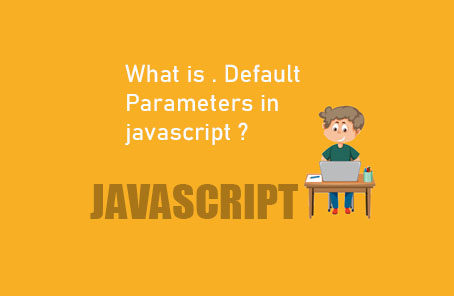
JavaScript introduced ES6 primarily to make our lives easier. One of the additions to achieve this is the default parameter feature. Many people expected such behavior from JavaScript earlier, and that’s why the default parameter was added.
If we want to set a default value for a parameter while defining a function, we can easily use ES6’s default parameters. However, in ES5, it wasn’t as straightforward. You can understand this by looking at the following examples.
First, we take a function with parameters and set default values for those parameters. If any arguments are provided while calling this function, it will use those argument values. If no arguments are provided, the default parameter values will be used. So in ES5, the code would look like this:
“`javascript
function defaultParams(name, age) {
name ? name = name : name = ‘Zonayed Ahmed’;
age ? age = age : age = 21;
console.log(‘My name is ‘ + name + ‘ and I am ‘ + age + ‘ years old!’);
}
“`
Now, if we call this function without any arguments:
“`javascript
defaultParams();
“`
The default values we set will be printed:
“`javascript
> defaultParams();
My name is Zonayed Ahmed and I am 21 years old!
“`
And if arguments are provided, the values of those arguments will be printed:
“`javascript
defaultParams(‘Zawad Ahmed’, 1);
“`
Now, the values of the arguments will be printed:
“`javascript
> defaultParams(‘Zawad Ahmed’, 1);
My name is Zawad Ahmed and I am 1 year old!
“`
Everything is fine, but it seems quite cumbersome, doesn’t it? Yes, that’s why default parameters were introduced in ES6. The same code in ES6 would look like this:
“`javascript
function defaultParams6(name = ‘Zonayed Ahmed’, age = 21) {
console.log(‘My name is ‘ + name + ‘ and I am ‘ + age + ‘ years old!’);
}
“`
Now, if we call this function without any arguments:
“`javascript
defaultParams6();
“`
The default values we set will be printed:
“`javascript
> defaultParams6();
My name is Zonayed Ahmed and I am 21 years old!
“`
And if arguments are provided:
“`javascript
defaultParams6(‘Zawad Ahmed’, 1);
“`
The values of the arguments will be printed:
“`javascript
> defaultParams6(‘Zawad Ahmed’, 1);
My name is Zawad Ahmed and I am 1 year old!
“`
**Default Parameters with Function Constructors**
Similarly, we can use default parameters with function constructors. But first, let’s look at the ES5 version of the code. In ES5, we had to do:
“`javascript
function FunctionConst(name, age) {
name ? name = name : name = ‘Zonayed Ahmed’;
age ? age = age : age = 21;
this.name = name;
this.age = age;
}
“`
Now, we will create a new object from this function constructor:
“`javascript
var zonayed = new FunctionConst();
“`
Now, if we check the name and age of this `zonayed`:
“`javascript
console.log(zonayed.name);
console.log(zonayed.age);
“`
The default parameters will be shown:
“`javascript
> console.log(zonayed.name);
Zonayed Ahmed
> console.log(zonayed.age);
21
“`
But if we define a new object with arguments:
“`javascript
var zawad = new FunctionConst(‘Zawad Ahmed’, 1);
“`
Now, if we check the name and age of this `zawad`:
“`javascript
console.log(zawad.name);
console.log(zawad.age);
“`
The values of the passed arguments will be shown:
“`javascript
> console.log(zawad.name);
Zawad Ahmed
> console.log(zawad.age);
1
“`
Similarly, if we write this function constructor in ES6:
“`javascript
function FunctionConst6(name = ‘Zonayed Ahmed’, age = 21) {
this.name = name;
this.age = age;
}
“`
Now, we will create two objects from this:
“`javascript
var zonayed6 = new FunctionConst6();
“`
Now, if we check the name and age of this `zonayed6`:
“`javascript
console.log(zonayed6.name);
console.log(zonayed6.age);
“`
The default parameters will be shown:
“`javascript
> console.log(zonayed6.name);
Zonayed Ahmed
> console.log(zonayed6.age);
21
“`
But if we define a new object with arguments:
“`javascript
var zawad6 = new FunctionConst6(‘Zawad Ahmed’, 1);
“`
Now, if we check the name and age of this `zawad6`:
“`javascript
console.log(zawad6.name);
console.log(zawad6.age);
“`
The values of the passed arguments will be shown:
“`javascript
> console.log(zawad6.name);
Zawad Ahmed
> console.log(zawad6.age);
1
“`
That’s it! Here, the ES6 style is much cleaner, easier, and looks better than the ES5 style.
—
Post Comment