What is Advance . Class in javascript?
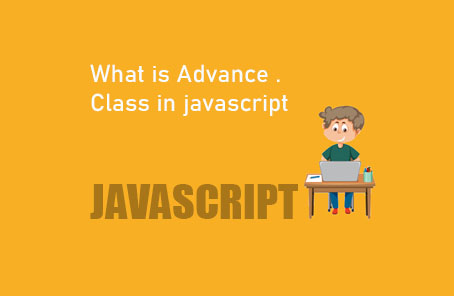
Until ES5, JavaScript did not have the concept of a class, unlike other programming languages. Those who come to JavaScript from other programming languages often get confused due to the absence of this feature and question whether JavaScript truly qualifies as an object-oriented programming language.
But the truth is, JavaScript is indeed an object-oriented language, although it is not class-based like other major languages; rather, it is a prototype-based language. That’s why JavaScript did not have the concept of classes until ES5. However, in ES6, classes were finally introduced as syntactic sugar.
In ES5, we could use function constructors in the following way:
“`javascript
var Child5 = function (name, dob) {
this.name = name;
this.dob = dob;
};
“`
Now we create a new object of this type as follows:
“`javascript
var zawad = new Child5(‘Zawad Ahmed’, 2017);
“`
If we want to print data from this object, we do it like this:
“`javascript
console.log(zawad.name);
console.log(zawad.dob);
“`
Output:
“`
Zawad Ahmed
2017
“`
Now, if we want to add a new method to our function constructor, we can easily do it like this:
“`javascript
Child5.prototype.currentAge = function() {
console.log(this.name + ‘ is ‘ + (2018 – this.dob) + ‘ years old!’);
};
“`
With this constructor function, all objects that are created can access this method:
“`javascript
zawad.currentAge();
> zawad.currentAge();
Zawad Ahmed is 1 years old! <undefined
“`
Now we will do the same thing using ES6 syntax, that is, using classes.
“`javascript
class Child6 {
constructor(name, dob) {
this.name = name;
this.dob = dob;
}
currentAge() {
console.log(`${this.name} is ${2018 – this.dob} years old!`);
}
}
“`
What? The syntax here doesn’t look familiar? If you have learned any other object-oriented programming language before, these should definitely look familiar. Here, the `constructor` method is used to set the initial values of your object. And in a class, this is a special method.
Anyway, now we will create a new object from this class:
“`javascript
const zawad6 = new Child6(‘Zawad Ahmed’, 2017);
“`
Now we can access the data from this new object in the same way:
“`javascript
console.log(zawad6.name);
console.log(zawad6.dob);
“`
Output:
“`
Zawad Ahmed
2017
“`
“In the same way, we can access the method inside the class:
zawad6.currentAge ();
› zawad6.currentAge();
Zawad Ahmed is 1 years old!
Now in JavaScript, classes can be declared in two ways:
1. Class Statement:
In a class statement, the class keyword is used for declaration. It is mandatory to provide a name for this type of class. The class used in the example above is read as follows:
“`javascript
class ClassStatement {
}
“`
2. Class Expression:
This works like function expressions. We take an anonymous class and assign it to a variable. Voilà, it becomes a class expression! The class name here is not necessary since ultimately we are assigning it to another variable. The variable carries the identity of the class. There is no difference between these two types of classes:
“`javascript
const ClassExpression = class {
“`
Now, in the case of classes, hoisting works a bit differently. Classes are not hoisted like functions, not even like function expressions. Many might mistakenly think that classes are hoisted, which is a logic error, similar to how function expressions are seen. However, unlike function statements or class statements, it might appear that way. But no! Classes are never hoisted. Hence, you cannot use a class before defining it.”
—
// First, we declare a constructor function using function expression
var Person5 = function(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
};
// Now, we want to assign a new method using prototype
Person5.prototype.dateOfBirth = function() {
console.log(this.name + ‘ born in ‘ + (2018 – this.age));
};
// Creating an object from the constructor function
var zawad5 = new Person5(‘Zawad Ahmed’, 7, ‘Child’);
// Now we can access the assigned method from the object
zawad5.dateOfBirth(); // Output: Zawad Ahmed born in 2011
Constructor Function (Person5):
- Person5 is a constructor function that takes name, age, and job parameters and assigns them to this.name, this.age, and this.job respectively.
Adding a Method (dateOfBirth):
- Person5.prototype.dateOfBirth adds a method dateOfBirth to the prototype of Person5. This means all instances created from Person5 will inherit this method.
Creating an Object (zawad5):
- var zawad5 = new Person5(‘Zawad Ahmed’, 7, ‘Child’); creates a new object zawad5 using the Person5 constructor.
Accessing the Method:
- zawad5.dateOfBirth(); calls the dateOfBirth method on the zawad5 object, which logs the name and calculates the birth year based on the age provided.
The provided JavaScript code demonstrates prototype-based inheritance in ES5. It defines a constructor function Person5 for creating objects with properties like name, age, and job. It then extends this constructor’s prototype with a method dateOfBirth, which calculates and logs the birth year based on the age provided when the object was instantiated.
By creating an object zawad5 from Person5 and calling zawad5.dateOfBirth(), you can access and utilize the dateOfBirth method to display information about the person’s birth year.
This approach showcases how JavaScript uses prototypes to implement inheritance and extend the functionality of objects created from constructor functions.
It looks like you’re trying to discuss how constructors and methods work in ES6 classes in JavaScript, and how you can define and access them. Let’s clarify and translate your provided code snippet into English for better understanding:
“`javascript
// We know, in ES6 class constructor method, we need to set our values inside. If we want, we can assign our methods outside as before. But we can also take the method if we want outside the constructor method as well:
class Person6 {
constructor(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
}
dateOfBirth() {
console.log(`${this.name} is born in ${2018 – this.age}`);
}
}
// Now, we can create an object as desired and everyone can access this method:
const zawad6 = new Person6(‘Zawad Ahmed’, 1, ‘Child’);
// Now we can access our said method from zawad6:
zawad6.dateOfBirth(); // Output: Zawad Ahmed is born in 2017
// Now we could also assign methods using ES5 techniques using prototype:
class Person56 {
constructor(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
}
}
“`
This code demonstrates the use of ES6 class syntax to define a `Person6` class with a constructor that initializes `name`, `age`, and `job` properties, as well as a `dateOfBirth` method that calculates and logs the birth year based on the given age. The example also shows how to create an instance (`zawad6`) of the `Person6` class and invoke the `dateOfBirth` method to print the person’s birth year.
Since we are claiming it as syntactic sugar, we are also working with the previous ES5 techniques:
“`javascript
Person56.prototype.dateOfBirth = function () {
console.log(this.name + ‘ born in ‘ + (2018 – this.age));
};
“`
Running this doesn’t produce any errors. Now, let’s create an object and see if we can access the method:
“`javascript
const zawad56 = new Person56(‘Zawad Ahmed’, 1, ‘Child’);
zawad56.dateOfBirth();
“`
From here, it’s clear that JavaScript hasn’t actually introduced a new object-oriented model, but rather it still supports all the previous ones. However, this practice of mixing ES5 and ES6 code is beneficial. Therefore, avoiding is
Post Comment