What is Class Inheritance in javascript ?
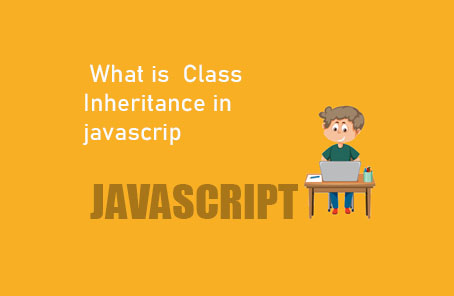
Now, if we encounter a situation where we may have two or more classes that are similar, as seen even in ES5 constructor functions, we can utilize inheritance. For example, let’s say we have a class called `PersonClassDemo`:
“`javascript
class PersonClassDemo {
constructor(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
this.dateOfBirth = () => {
console.log(`${this.name} is born in ${2018 – this.age}`);
};
}
}
“`
And another class called `TeacherClassDemo`, which shares most properties with `PersonClassDemo` but has one additional property:
“`javascript
class TeacherClassDemo extends PersonClassDemo {
constructor(name, age, job, dateOfBirth, subject) {
super(name, age, job);
this.subject = subject;
this.dateOfBirth = dateOfBirth;
}
}
“`
In this case, we don’t need to create two separate classes from scratch. We can inherit the properties and methods from the `PersonClassDemo` into `TeacherClassDemo`. Assume we have the first class as follows:
“`javascript
class PersonClass {
constructor(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
}
“`javascript
“Now let’s create another one for the teacher, which will have all the characteristics of a person, plus an additional one. We will inherit everything and define the extra one like this:
“`javascript
class TeacherClass extends PersonClass {
constructor(name, age, job, subject) {
super(name, age, job);
this.subject = subject;
}
}
“`
Great, our inheritance is set up. Here we have used a special keyword `super`. Everything else remains the same. We have defined what should be in the constructor method, and then used the `super` keyword to inherit those from `PersonClass` (here `name`, `age`, `job`). That’s how inheritance works. Now let’s create a new object:
“`javascript
const ourSir = new TeacherClass(‘Shafiq Sir’, 46, ‘Assistant Teacher’, ‘Physics’);
“`
Now that the new object is created, let’s test if everything is correct:
“`javascript
console.log(ourSir.name);
console.log(ourSir.age);
console.log(ourSir.job);
console.log(ourSir.subject);
“`
Output should be:
“`
Shafiq Sir
46
Assistant Teacher
Physics
“`
Similarly, if our class has any methods, they will also be inherited easily. Let’s say we have a class with methods:
“`javascript
class PersonClassMeth {
constructor(name, age, job) {
this.name = name;
this.age = age;
this.job = job;
}
dateOfBirth() {
console.log(`${this.name} born in ${2018 – this.age}`);
}
}
“`
Now let’s create another class that inherits everything:
“`javascript
class TeacherClassMeth extends PersonClassMeth {
constructor(name, age, job, subject) {
super(name, age, job);
this.subject = subject;
}
}
“`
Actually, we don’t need to do anything extra to bring methods from the parent class; the `super` keyword will handle everything for us. Now let’s create an object from this class:
“`javascript
const ourSirMeth = new TeacherClassMeth(‘Shafiq Sir’, 46, ‘Assistant Teacher’, ‘Physics’);
“`
Now we can access all other properties and also access our method:
“`javascript
console.log(ourSirMeth.dateOfBirth());
“`
Output should be:
“`
Shafiq Sir born in 1972
“`
Now, we may want some methods that exist only in our class and not accessible to objects created from the class. Yes, we can take such methods using a special keyword `static`:
“`javascript
class StaticMethod {
constructor(name) {
this.name = name;
}
static aSpecMeth() {
console.log(‘I am A Special Method!’);
}
}
“`
Now we can directly access this method via the class:
“`javascript
console.log(StaticMethod.aSpecMeth());
“`
Output should be:
“`
I am A Special Method!
“`
However, if we create an object from this class:
“`javascript
const aMeth = new StaticMethod(‘Zonayed Ahmed’);
“`
And then try to access the static method like this:
“`javascript
console.log(aMeth.aSpecMeth());
“`
We will get an error:
“`
Uncaught TypeError: aMeth.aSpecMeth is not a function
“`
Because it’s a static method. It can only be accessed through the class itself, not through objects created from the class.
One more thing to note, the code inside the class will run in strict mode.”
“`
Post Comment