First-Class Functions and Higher-Order Functions in javascript
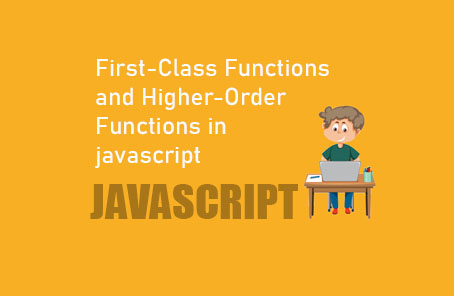
In JavaScript, functions are called first-class functions. So, what is a first-class function? A first-class function can be passed as an argument to another function, a function can return another function, and most interestingly, a function can be stored as a variable.
### Passing a Function as an Argument:
“`javascript
function callMyName(name, callback) {
var myAge = 28;
callback(myAge);
console.log(‘Is it interesting? Yes it is Mr.’ + name);
}
function hello(age) {
console.log(‘I am passed through argument and my age is: ‘ + age);
}
callMyName(‘Zonayed Ahmed’, hello);
“`
**Output:**
“`
I am passed through argument and my age is: 28
Is it interesting? Yes it is Mr.Zonayed Ahmed
“`
### Returning a Function from a Function:
“`javascript
function welcomeMsg(name) {
console.log(‘Welcome Mr. ‘ + name);
return function options(menu) {
console.log(‘Do you like ‘ + menu + ‘ Mr. ‘ + name);
};
}
welcomeMsg(‘Zonayed Ahmed’)(‘Coffee’);
“`
**Output:**
“`
Welcome Mr. Zonayed Ahmed
Do you like Coffee Mr. Zonayed Ahmed
“`
—
This explanation demonstrates the concept of first-class functions and higher-order functions in JavaScript, with examples of passing functions as arguments and returning functions from other functions.
—
**Storing a function as a variable:**
“`javascript
var aFunc = function(name) {
console.log(‘I am simply a function and my name is ‘ + name);
};
var anotherVar = aFunc;
anotherVar(‘Zonayed Ahmed’);
“`
Now, what is the benefit of a function being first-class? Yes, these are useful in many ways as we can use functions in various ways. Additionally, we know about functional programming. In functional programming, higher-order functions are used where the concept of first-class is very important. In higher-order functions, we can pass one function as an argument to another function, and we can return a function from another function. All of these ideas come from the concept of first-class functions. Therefore, we can say that first-class functions are very important for functional programming.
### 01. `map()`, `filter()`, and `reduce()`
These three methods are the most commonly used nowadays. These three methods perform almost the same type of work. They are methods of arrays, and their main task is to apply a function to each item of the applied array. They work like a loop but are not as meaningless or complex as a loop. It might be a bit difficult to understand at first, but once you get the hang of it, you will find them to be the best.
If you write in the Chrome console:
“`javascript
console.dir(Array.prototype)
“`
You will find many methods inside the array prototype, including these three methods:
– `fill: ƒ fill()`
– `filter: ƒ filter()`
– `find: ƒ find()`
– `findIndex: ƒ findIndex()`
– `flat: ƒ flat()`
– `flatMap: ƒ flatMap()`
– `forEach: ƒ forEach()`
– `includes: ƒ includes()`
– `indexOf: ƒ indexOf()`
– `join: ƒ join()`
– `keys: ƒ keys()`
– `lastIndexOf: ƒ lastIndexOf()`
– `length: 0`
– `map: ƒ map()`
– `pop: ƒ pop()`
– `push: ƒ push()`
– `reduce: ƒ reduce()`
– `reduceRight: ƒ reduceRight()`
—
The methods mentioned here, such as `map()` and `reduce()`, take a callback function as the first argument, and the second argument sets what the value of `this` will be inside the callback function. For example, in the case of `map()`:
“`javascript
anArray.map(callbackFunction, thisArg);
“`
However, remember that the argument that determines the value of `this` behaves slightly differently in arrow functions due to the lexical `this` keyword in arrow functions. This feature isn’t used very often, but it’s good to be aware of its existence.
`map()`:
When you call `map()` on an array, it applies a given function to each item of the array and returns a new array with the results.
Suppose you have an array:
“`javascript
var arr = [1, 2, 3, 4, 5, 6, 7, 8, 9];
“`
Now, if you want to get the square of each item in this array, you could do:
“`javascript
var anotherArr = [];
for (var i = 0; i < arr.length; i++) {
anotherArr.push(arr[i] * arr[i]);
}
“`
In `anotherArr`, you will get your desired results. Although this is correct, notice that we had to create a new array. Additionally, we had to use another method, `push()`. And inside the `for` loop, we are using several things that are somewhat redundant, and if you accidentally use a colon or comma instead of a semicolon, the code will not work.
You can achieve the same result using `map()`. First, declare the function you want to apply to each item. We want to get the square of each item:
“`javascript
function getSquare(item) {
return item * item;
}
“`
“`javascript
// Now you want to apply this function to each item in your array. This is where map() comes in.
arr.map(getSquare);
// Now we know, it also returns an array. Now that array needs to be stored somewhere:
var newArr = arr.map(getSquare);
// See the result:
console.log(newArr);
// > console.log(newArr);
// ► (9) [1, 4, 9, 16, 25, 36, 49, 64, 81]
// Now the whole thing can be written like this:
var newArr = arr.map(function (item) {
return item * item;
});
// This whole thing will give the same result as before. Now here you will see that we have used an argument called item. But here map() accepts three arguments. The first one, which we saw, represents each item of the array, the second argument is the index number of the array item, and the third argument always gives the full array.
var newArr = arr.map(function (item, index, fullArr) {
console.log(‘Item: ‘ + item + ‘ and index: ‘ + index + ‘. Full Array: ‘ + fullArr);
});
// Result:
Item: 1 and index: 0. Full Array: 1,2,3,4,5,6,7,8,9
Item: 2 and index: 1. Full Array: 1,2,3,4,5,6,7,8,9
Item: 3 and index: 2. Full Array: 1,2,3,4,5,6,7,8,9
Item: 4 and index: 3. Full Array: 1,2,3,4,5,6,7,8,9
Item: 5 and index: 4. Full Array: 1,2,3,4,5,6,7,8,9
Item: 6 and index: 5. Full Array: 1,2,3,4,5,6,7,8,9
Item: 7 and index: 6. Full Array: 1,2,3,4,5,6,7,8,9
Item: 8 and index: 7. Full Array: 1,2,3,4,5,6,7,8,9
Item: 9 and index: 8. Full Array: 1,2,3,4,5,6,7,8,9
“`
### Map():
If you use ES6 syntax like this:
“`javascript
const newArr6 = arr.map(item => item * item);
“`
It will produce the same result. See how concise and elegant the code is.
### Filter():
Filter works similar to map, but here, if the applied function returns true, the item will be included in the new array; otherwise, it won’t. Suppose I want to extract only even numbers from the following array:
“`javascript
var arr = [1, 2, 3, 4, 5, 6, 7, 8, 9];
“`
Now, applying the filter method makes it easy:
“`javascript
var newArr = arr.filter(function(item) {
return item % 2 === 0;
});
“`
Here, the function is applied to each item. Items that return true are placed in the new array, while items that return false are not. Just like map, filter also accepts three arguments:
“`javascript
var newArr = arr.filter(function(item, index, fullArr) {
console.log(‘Item: ‘ + item + ‘ and index: ‘ + index + ‘. Full Array: ‘ + fullArr);
});
“`
You can also use anonymous or external functions as with map. See the result:
“`javascript
console.log(newArr);
// Output: [2, 4, 6, 8]
“`
### Using ES6 Syntax
When using ES6 syntax, you can filter an array and use the `reduce()` method:
“`javascript
const newArrFilter6 = arr.filter(item => item % 2 === 0);
“`
### Using `reduce()`
The `reduce()` method, unlike `map()` or `filter()`, takes an extra argument. In programming, we understand the concept of a state variable, which keeps track of specific data during operations. Simply put, if we want to find the total of all numbers in an array, we can easily achieve that using `reduce()`. For example, if we have an array:
“`javascript
var arr = [1, 2, 3, 4];
“`
To find the total sum of all items in the array, we can use `reduce()`:
“`javascript
var total = arr.reduce(function(sum, item) {
return sum + item;
}, 0);
// Now, ‘total’ will be 10
“`
In this example, `reduce()` is perfect for operations where maintaining a state variable is ideal, such as calculating the total of all array items.
It seems like you’ve provided a code snippet that involves using the `reduce` method in JavaScript to calculate the total sum of an array. The `reduce` method iterates through each item of the array and accumulates a single value (in this case, the sum of all elements).
“`javascript
// Using var (ES5 syntax)
var total = arr.reduce(function(sum, item, index, fullArr) {
console.log(‘Item: ‘ + item + ‘ and index: ‘ + index + ‘. Full Array: ‘ + fullArr + ‘ and sum: ‘ + sum);
return sum += item;
}, 0);
// Using const with arrow function (ES6+ syntax)
const total = arr.reduce((sum, item) => sum += item, 0);
“`
In both cases (`var` and `const` with arrow function), the `reduce` method starts with an initial value of `0` (`sum`), iterates through each element (`item`) in the array (`arr`), and accumulates the sum (`sum += item`). Finally, it returns the total sum of all elements in the array. This method is powerful for reducing an array to a single value based on some computation logic.
It seems like you’re discussing JavaScript array operations and the behavior of the `this` keyword within different contexts such as regular functions and arrow functions.
—
Let’s say we want to perform some operations on an array. I have taken a basic array:
“`javascript
const anoArray = [1, 2, 4, 5, 6, 7, 9, 3];
“`
Now, let’s use `map()` on it to see what value `this` holds inside the callback function:
“`javascript
anoArray.map(function (single, index, fullArr) {
console.log(this);
});
console.log(this);
“`
When this runs, in the console you’ll see:
“`
▸ window [postMessage: f, blur: f, focus: f, …]
* (8) [undefined, undefined, undefined, undefined, undefined, undefined, undefined, undefined]
“`
Clearly, `this` here indicates the global object or `window`. Now, to determine the value of `this` as our argument, we’ll use an object `anObj` that we’ve created:
“`javascript
anoArray.map(function (single, index, fullArr) {
console.log(this);
}, anObj);
“`
This will run and now in the console you’ll see:
“`
{ name: “Zonayed Ahmed”, age: 21 }
“`
We have changed the value of `this` inside our callback function. The same won’t work in the case of an arrow function because arrow functions have lexical `this` binding. This is discussed in detail in the ES6 chapter.
Post Comment