The some() and every() methods
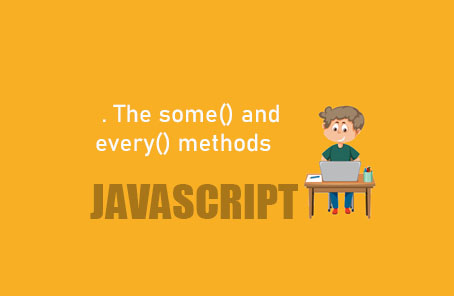
are generally used when there is multiple data in an array. These methods help us filter or operate on data within the array as needed. Similar to the previously discussed map, filter, and reduce methods, there are two more useful methods: some() and every(). These methods share similarities with the earlier map and filter methods. We might encounter situations where we need to check if a specific data is present in an array. For instance, we have an array like this: const arr = [1, 2, 4, 5, 6, 7, 8]; Now, we want to know if this array contains the number 7. Or, we might need to check if every data within the array follows a specific pattern. In such cases, these two methods can be very useful. Both methods accept two arguments. The first one is a callback function, and the second one determines the value of ‘this’ inside the callback function. The first argument, i.e., the callback function, is mandatory. However, the argument for determining the value of ‘this’ is optional. If not provided, ‘this’ will behave by default. But if you want to change it, you can provide this argument. anArr.some(callback, thisArg); anArr.every(callback, thisArg); Here, you can work with ‘this’ just like in the previous map and filter methods. However, I will mainly show what these two methods, some() and every(), actually do. These some() and every() methods always return either true or false. The some() method works exactly as its name suggests. Given an array, this method answers whether there are some values (one or more) in the array that match a certain condition. For example, we have an array: const anArrSome = [‘hello’, 1, 2, 3, ‘Bangla’, 5, ‘world’, 7, 8, 9];”””
Now, is there a 9 in this array? Let’s see using some() anArrSome.some(function (anElement) { }) return anElement 9 If you run this, it will return true because we do have a 9 in our array:
anArrSome.some(function (anElement) { return anElement = 9 }) <true Now, let’s see if there is a 10: anArrSome.some(function (anElement) { return anElement — 10 }) This will return false because we do not have a 10 in our array: anArrSome.some(function (anElement) { return anElement === 10 }) <false Now, to explain this further, the inner callback function will run once for each item in the array. The callback function accepts three arguments. The first argument is the current item of the array that the callback function is running on, the second argument is its index number, and the third argument gives access to the entire array. anArr.some(function (current Element, index, theArray) { // some codes // return true or false.
—
If we use ES6 syntax:
“`javascript
anArr.some((currentElement, index, theArray) => {
// some codes
// return true or false
});
“`
We can look at the following example to understand which argument serves which purpose:
“`javascript
anArrSome.some((currentElement, index, theArray) => {
console.log(‘Item: ‘ + currentElement + ‘ and index: ‘ + index + ‘. Full Array: ‘ + theArray);
});
“`
– The first argument is the current element.
– The second argument is the index number.
– The last argument is the full array.
For example:
“`
Item: hello and index: 0. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 1 and index: 1. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 2 and index: 2. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 3 and index: 3. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: Bangla and index: 4. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 5 and index: 5. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: world and index: 6. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 7 and index: 7. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 8 and index: 8. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
Item: 9 and index: 9. Full Array: hello,1,2,3,Bangla,5,world,7,8,9
false
“`
Another important aspect of the `some()` method is that it stops executing once it finds an element that meets the condition and returns `true`. It doesn’t check the remaining elements. Look at the following example:
“`javascript
anArrSome.some(anElement => {
console.log(“Current Element: ” + anElement);
return anElement === 1;
});
“`
If you run this, the output will be:
“`
Current Element: hello
Current Element: 1
true
“`
—
This explanation helps you understand the use of `some()` in ES6, including how it handles arguments and stops iterating once a condition is met.
“`markdown
Look, it checked the first one, which returned false. So, it went to the next one, which actually met our condition, and hence it returned true. Now, this method will return true and stop. We can see this exactly in the output.
Now, we know about a special statement, which is the `break` statement. Its job is to stop a loop when a certain condition is met. If you’re not familiar with it, look at the following example:
“`javascript
var anArr = [1, 2, 4, 5, 6, 7, 8, 9];
for (var i = 0; i < anArr.length; i++) {
console.log(‘Current Value: ‘ + anArr[i]);
if (anArr[i] === 5) {
break;
}
}
“`
Here, we are running a loop on an array. Now, if the element of our array is 5, we will exit the loop. For this, we used a condition with the `break` statement so that exactly when 5 comes, we will exit the loop. This is how the program will work:
“`javascript
> var anArr = [1, 2, 4, 5, 6, 7, 8, 9];
for (var i = 0; i < anArr.length; i++) {
console.log(‘Current Value: ‘ + anArr[i]);
if (anArr[i] === 5) {
break;
}
}
Current Value: 1
Current Value: 2
Current Value: 4
Current Value: 5
< undefined
“`
Now, you cannot use this `break` statement in `map()`, `filter()`, `reduce()`, `some()` or such methods. But there might come a time when you need to stop such a loop or iteration. So, we can do this a bit differently with the `some()` method:
“`javascript
anArr.some(anElement => {
console.log(‘Current value: ‘ + anElement);
return anElement === 5;
});
“`
“See, this is just like our upper loop, it breaks the iteration whenever it gets 5:
“`javascript
anArr.some (anElement => {
console.log(`Current value: ${anElement}`);
return anElement === 5;
});
“`
Output:
“`
Current value: 1
Current value: 2
Current value: 4
Current value: 5
<true
“`
So, in such special cases, this kind of method can be very useful. We can use this method for an array of objects. Suppose we have an array of such objects:
“`javascript
const objSome = [
{
name: ‘Zawad Ahmed’,
age: 1,
job: ‘Child’
},
{
name: ‘Zobayer Ahmed’,
age: 31,
job: ‘Pharmacist’
},
{
name: ‘Zonayed Ahmed’,
age: 21,
job: ‘Student’
}
];
“`
Now suppose we want to check if any item here has a property named `age`:
“`javascript
objSome.some(anObj => anObj.age);
“`
This will return true:
“`javascript
> objSome.some(anObj => anObj.age)
true
“`
—
Now, suppose we want to check if there is anyone who is 1 year old among all the data present here:
“`javascript
objSome.some(anObj => anObj.age === 1)
“`
This will also return true:
“`javascript
> objSome.some(anObj => anObj.age === 1)
true
“`
Now, let’s assume we want to check if there is a teacher here:
“`javascript
objSome.some(anObj => anObj.job === ‘Teacher’)
“`
But if there is no teacher here, it will return false:
“`javascript
> objSome.some(anObj => anObj.job === ‘Teacher’)
false
“`
### `every()` Method
This method works similarly to the previous ones, but in this case, it checks if every item in your array meets the given condition. It will only return true if every item meets the condition. If even one item fails to meet the condition, it will return false.
Let’s start with a very simple example. Assume we have an array like this:
“`javascript
const arrEvery = [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1];
“`
Now, is every item here equal to 1?
“`javascript
arrEvery.every(function(anItem) {
return anItem === 1;
})
“`
This will return true, since every item here is 1:
“`javascript
> arrEvery.every(function(anItem) { return anItem === 1; })
true
“`
—
“`javascript// Using ES6 syntax
arrEvery.every(anItem => anItem === 1);
// Now, if even one item was something other than 1, it would return false. Let’s assume we have another array that looks the same but has one different item.
const arrDiffEvery = [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2];
// Now, let’s check if all the items in this array are 1
arrDiffEvery.every(anItem => anItem === 1);
// It will simply return false since although all items are the same, one item (the last one) is different:
> arrDiffEvery.every(anItem => anItem === 1); // false
// Now, this method also takes three arguments just like the previous ones.
anArr.every((currentElement, index, theArray) => {
// codes
// should return true or false
});
// The first one is the element of the array on which the callback is running, the second one is its index number in the array, and the last one is the whole array:
arrDiffEvery.every((currentElement, index, theArray) => {
console.log(‘Item: ‘ + currentElement + ‘ and index: ‘ + index + ‘. Full Array: ‘ + theArray);
return true;
});
“`
—
“`text
Look at what this argument prints:
1,2
Item: 1 and index: @. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 1 and index: 1. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 1 and index: 2. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 1 and index: 3. Full Array: 1,1,1,1,1,1,1,1,1
Item: 1 and index: 4. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 1 and index: 5. Full Array: 1,1,1,1,1,1,1,
Item: 1 and index: 6. Full Array: 1,1,1,1,1,1,1,1,1
Item: 1 and index: 7. Full Array: 1,1,1,1,1,1,1,
Item: 1 and index: 8. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 1 and index: 9. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 1 and index: 10. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Item: 2 and index: 11. Full Array: 1,1,1,1,1,1,1,1,1,1,1,2
Now, this `every` is exactly like `some`, since if any condition is false, it will return false for the entire array. So, as soon as it encounters a false condition, the loop or iteration stops. Let’s assume we have another array below:
“`javascript
const anNumberArrEvery= [1, 1, 1, 3, 4, 5, 6, 7, 8, 9];
“`
Now we will see if all the elements here are 1:
“`javascript
anNumberArrEvery.every (anElement => {
console.log(“${anElement} is printed”);
return anElement == 1;
});
“`
Now, when you run this, you will see that it stops exactly when it returns false:
“`
1 is printed
3 is printed
false
“`
So, in the same way, we can use the mechanism of the break statement here. We can also use this method for various other purposes. For example, now we want to check if all items in our above array are numbers:
“`javascript
anNumberArrEvery.every (anElement => typeof anElement === ‘number’);
“`
This will return true since all items in this array are numbers:
“`javascript
anNumberArrEvery.every (anElement => typeof anElement === ‘number’); // true
In this way, we can use this method in many different ways. For example, we can use it with objects as well. Let’s say we have an array of objects like this:
“`javascript
const objEvery = [
{ name: ‘Zawad Ahmed’, job: ‘Child’ },
{ name: ‘Zobayer Ahmed’, age: 31, job: ‘Pharmacist’ },
{ name: ‘Zonayed Ahmed’, age: 21, job: ‘Student’ }
];
“`
Now we want to know if each object in the array has the `name` property:
“`javascript
objEvery.every(anObj => anObj.name);
“`
This will return `true` since every object here has the `name` property:
“`javascript
> objEvery.every(anObj => anObj.name);
true
“`
Now, if we want to see if each object has the `age` property:
“`javascript
objEvery.every(anObj => anObj.age);
“`
This will return `false` since not every object here has the `age` property:
“`javascript
> objEvery.every(anObj => anObj.age);
false
“`
Post Comment