How to Run loop on object in javascript ?
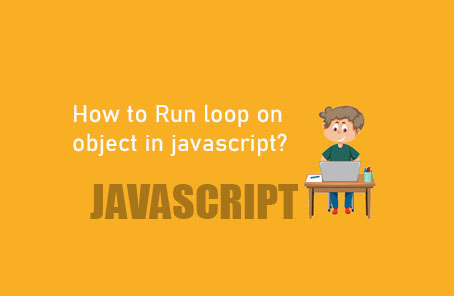
“`javascript
// Object iteration using for loop
// Suppose we have an object like this:
var obj = {
Bangladesh: ‘Dhaka’,
India: ‘Delhi’,
Nepal: ‘Kathmandu’,
Afghanistan: ‘Kabul’,
Thailand: ‘Bangkok’
};
// Now, in a situation like this, we might want to list all the names or just the capitals.
// This can be easily done whether it’s an array or an object using a for loop or some other method.
// Here, you can iterate over the object using a for loop like this:
for (var name in obj) {
console.log(name); // This will print just the names
}
// And using these names, we can easily retrieve their values:
for (var name in obj) {
console.log(obj[name]);
}
“`
This code snippet demonstrates how to iterate over the keys (names) of an object (`obj`) using a `for…in` loop in JavaScript and print them. It also shows how to access the corresponding values of each key in the object.
“`plaintext
Although everything can be done this way, JavaScript developers prefer functional programming. However, if we need to take all values or an array, it may cause a little trouble here.
I now want to print the capitals of all countries. Now we can see the methods of the Object constructor here. Each object created here has an Object constructor.
> for (name in obj) { console.log(obj[name]);
Dhaka Delhi
Kathmandu
Kabul Bangkok
> obj.constructor === Object
true
We can also use obj.constructor directly or use the direct Object constructor. Now inside this constructor, there are some methods that we can see:
console.dir(Object);
● is frozens of is frozen () ►isSealed: f isSealed() keys: f keys()
length: 1
name: “Object”
▸ preventExtensions: f preventExtensions()
prototype: {constructor: f, defineGetter_ f, define Sett
▸ seal: f seal()
setPrototypeof: f setPrototype of()
▸ values: f values()
proto_f()
★ [[Scopes ]]: Scopes[e]
Here you will find two methods named keys and values.
Now if we want to get the values of our object, we need to use the method named values here. It will accept the object as an argument and return all values as an array at the end.
Object.values(obj);
> Object.values(obj)
(5) [“Dhaka”, “Delhi”, “Kathmandu”, “Kabul”, “Bangkok”]
“`
This translation captures the essence of the text, explaining various JavaScript concepts related to object constructors, methods like `Object.values()`, and iterating through object properties.
“`javascript
// Storing the returned array in a variable
var capitals = Object.values(obj);
// Logging the contents of the ‘capitals’ array
console.log(capitals);
// Output:
// [“Dhaka”, “Delhi”, “Kathmandu”, “Kabul”, “Bangkok”]
// Using a loop to iterate through the ‘capitals’ array
capitals.map(function(capital) {
console.log(‘Capital is: ‘ + capital);
});
// Output:
// Capital is: Dhaka
// Capital is: Delhi
// Capital is: Kathmandu
// Capital is: Kabul
// Capital is: Bangkok
// Performing the entire operation in one line
Object.values(obj).map(function(capital) {
console.log(‘Capital is: ‘ + capital);
});
// Output (same as above):
// Capital is: Dhaka
// Capital is: Delhi
// Capital is: Kathmandu
// Capital is: Kabul
// Capital is: Bangkok
“`
This code snippet demonstrates how to store the values of an object in an array (`capitals`), iterate over the array using `.map()`, and log each capital city with a prefixed message “Capital is: “.
:
“`javascript
// Using ES6 syntax:
Object.values(obj).map(capital => console.log(`Capital is ${capital}`));
// Output will be the same as before, focusing on values only.
// If we also need the keys, then we can use the keys method.
// In our example object, if we want to print both the country names and capitals,
// the keys method will work similar to the values method. However, in this case,
// keys will return the keys. Then, later, we can use these keys to find the values as well.
// That means both keys and values can be used.
Object.keys(obj);
// (5) [“Bangladesh”, “India”, “Nepal”, “Afghanistan”, “Thailand”]
// Now we can store this new array somewhere for later use.
var countries = Object.keys(obj);
// Now, when we log this to the console:
console.log(countries);
// (5) [“Bangladesh”, “India”, “Nepal”, “Afghanistan”, “Thailand”]
// From here, we can also work with map:
countries.map(function(country) {
console.log(‘Country Name: ‘ + country);
});
“`
Output:
“`
Country Name: Bangladesh
Country Name: India
Country Name: Nepal
Country Name: Afghanistan
Country Name: Thailand
“`
This code demonstrates how to use `Object.keys` to retrieve the keys of an object (in this case, country names), and then use `map` to iterate over these keys and print each country name.
Post Comment