What is Dot Notation and Brackets Notation in javascript ?
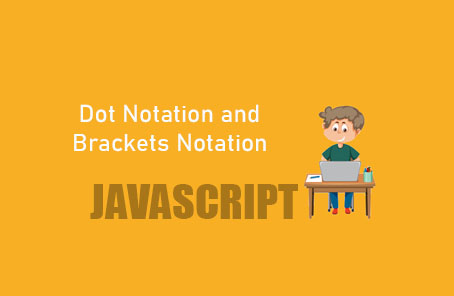
When we need to access a property from an object in JavaScript, we can do it in two ways:
– Dot Notation
– Brackets Notation
First, let’s look at an example to understand where exactly we use them. Suppose we have an object:
“`javascript
const obj = {
property: ‘value’
};
“`
Now, if we want to access the property from this object using dot notation:
“`javascript
obj.property
“`
And if we use brackets notation:
“`javascript
obj[‘property’]
“`
So, if we access and print it in both ways:
“`javascript
console.log(obj.property);
console.log(obj[‘property’]);
“`
Both will give the same output:
“`
> console.log(obj.property);
console.log(obj[‘property’]);
value value
“`
Now, if both give the same output, what is the need for having both? Wouldn’t one be enough? But no! While dot notation is used most of the time, there are situations where brackets notation is also useful. However, one thing to note is that where dot notation works, it is conventionally preferred (a favorite among developers).
—### Dot Notation:
Let’s say we have an object:
“`javascript
const person = {
name: ‘Zonayed Ahmed’,
age: 21,
job: ‘Student’
}
“`
Now, if we want to access the `name` property from this `person` object, we need to use dot notation like this:
“`javascript
person.name
“`
Here, `person` is our object, then a dot (`.`) means inside it, followed by `name`, which is the name of the property. In short, we are trying to access a property named `name` inside the `person` object. So, if we print this:
“`javascript
console.log(person.name);
“`
We will see that the value of the `name` property of the `person` object comes out. The value here is `Zonayed Ahmed`.
Now, suppose you can’t directly access the property name or the key of the object but instead need to access it in some other way, like as a variable:
“`javascript
const personName = ‘name’;
“`
Now you need to use this variable to access the `name` property from your `person` object. It seems simple, right? You might think this would work:
“`javascript
person.personName
“`
Let’s see what happens if we print this:
“`javascript
console.log(person.personName);
“`
But this will output:
“`javascript
undefined
“`
—
Even though dot notation works in this situation, it is better to use dot notation. Because dot notation looks good, and the code appears much cleaner.
Now, let’s look at some more uses of bracket notation. Suppose we have another object:
“`
const anyObj = {
Student: ‘A property name to match with the previous one’
};
“`
Here, I have named the property of `anyObj` in this way because, in our previous `person` object, there is a property value `Student`. We want to see if we can access the `Student` property here using that value:
“`
anyObj[person.job]
“`
Here, we are trying to access a property from `anyObj` using bracket notation. What is that property? It is `person.job`. Now, we need to see what the value of `person.job` is. The value of `person.job` is `Student`. That means we are actually looking for the value of a property named `Student` in `anyObj`:
“`
console.log(anyObj[person.job]);
“`
And just as expected, it works:
“`
console.log(anyObj[person.job]); // Output: A property name to match with the previous one
“`
Now, we know how dot notation works. In most cases, we will work with dot notation. But when should we use bracket notation? Yes, if we want to access all property values of an object using a `for` loop, then bracket notation is useful. Suppose we want to print all property values of the above `person` object:
“`
for (aval in person) {
console.log(`aval is here: ${aval}`);
}
“`
This will output:
“`
aval is here: name
aval is here: age
aval is here: job
“`
See, by using this loop, we are getting the property keys. But how can we get the values then? Yes, by using bracket notation with these keys, we can also get the values like this:
}
for (aval in person) {
console.log(‘Value: ${person[aval]}’);
Absolutely perfect
> for (aval in person) {
}
console.log(‘Value: ${person[aval]}’);
Value: Zonayed Ahmed
Value: 21
Value: Student
If we try this with dot notation, it won’t work:
for (aval in person) {
console.log(‘Value: ${person.aVal}’);
Here you’ll see the same undefined.
> for (aval in person) {
}
console.log(‘Value: ${person.aval}’);
Value: undefined
Post Comment