What is Logical Operators and (&&) in javascript ?
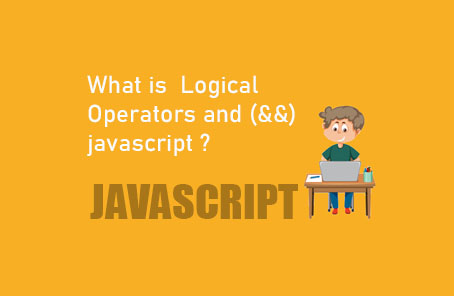
This && operator is used between two expressions:
‘Go to the market’ && ‘Do the shopping’
Now, notice that you can only do the shopping if you go to the market: ‘Go to the market’ and ‘Do the shopping’.
The AND operator works in exactly the same way. If your first expression is true, only then will the second one execute.
And if the first one is false, then the second one will never execute:
“`javascript
console.log(true && false);
// Output: false
console.log(true && true);
// Output: true
console.log(false && true);
“`
Even if the second one is true, it doesn’t matter, because the first one is false, and hence, it won’t be considered:
“`javascript
console.log(false && true);
// Output: false
“`
In this way, we can use this technique to perform more advanced tasks. We can move to the next action depending on the first expression. Meaning, if the first one is true, only then will the next one execute:
“`javascript
true && console.log(‘Eat’);
“`
“`
“See, here ‘Eat’ has been printed. Because the first expression is true:
“`javascript
true && console.log(‘Eat’);
“`
Output:
“`
Eat
“`
But if the first one is false:
“`javascript
false && console.log(‘Eat’);
“`
`Eat` will not be printed because the first value is false:
“`javascript
false && console.log(‘Eat’); // false
“`
The same applies even if it’s assigned to a variable:
“`javascript
const anoVar = ‘Me’ && ‘You’;
console.log(anoVar);
“`
Output:
“`
You
“`
Why did this happen? Yes, because the first one was true, it assigned the second one. Thus, the second one was stored in the variable `anoVar`. Now, if the first one is false, the second one will not be considered:
“`javascript
const anoVar2 = false && ‘Anything’;
console.log(anoVar2);
“`
Output:
“`
false
“`
—
This explanation highlights how JavaScript’s logical AND (`&&`) operator works based on truthy and falsy values.
“`javascript
// First, we print if the ‘name’ property exists in the object.
const userAnd = {
name: ‘Zonayed Ahmed’
};
if (userAnd.name) {
console.log(`Name is: ${userAnd.name}`);
}
// Using the && operator technique for the same output.
userAnd.name && console.log(`Name is: ${userAnd.name}`);
// Now, let’s print another property (‘age’) if it exists in the object.
if (userAnd.age) {
console.log(`Age is: ${userAnd.age}`);
}
“`
This code checks if the `name` property exists in the `userAnd` object and prints it if true, using both `if` statements and the short-circuiting `&&` operator. It also demonstrates how to extend this logic to check and print the `age` property if it exists.
1. Original:
“`
> if(userAnd.age) {
}
console. log (‘Age is: S (userAnd.age}’ );
undefined
“`
Translated:
“`
if (userAnd.age) {
console.log(`Age is: ${userAnd.age}`);
}
“`
2. Original:
“`
> userAnd.age && console.log(“Age is: $[userAnd.age}”); undefined
“`
“`
userAnd.age && console.log(`Age is: ${userAnd.age}`);
“`
3. Original:
“`
> userAnd.name && userAnd.age && console.log(“$(userAnd.name) ${userAnd.age) years old!’);
“`
Translated:
“`
if (userAnd.name && userAnd.age) {
console.log(`${userAnd.name} ${userAnd.age} years old!`);
}
“`
4. Original:
“`
> userAnd.name && userAnd.age && console.log(“${userAnd.name) is $[userAnd.age) years old!”);
undefined
“`
Translated:
“`
userAnd.name && userAnd.age && console.log(`${userAnd.name} is ${userAnd.age} years old!`);
“`
5. Original:
“`
> (userAnd.name userAnd.age) && console.log(“Found: $(userAnd. name || userAnd.age)’);
“`
Translated:
“`
(userAnd.name || userAnd.age) && console.log(`Found: ${userAnd.name || userAnd.age}`);
“`
These translations should now correctly reflect the intent of each JavaScript statement, using template literals where appropriate for string interpolation.
“In JavaScript, we can perform tasks using expressions as we wish. Similarly, functions can be evaluated based on their truth or falsehood. Suppose we have a function like this:
“`javascript
const anoFunc = () => {
console.log(‘Print Me!’);
}
“`
Now, based on an expression, we can conditionally call this function:
“`javascript
true && anoFunc();
“`
Here, the function will execute:
“`
> true && anoFunc();
Print Me!
“`
If it’s false:
“`javascript
false && anoFunc();
“`
The function won’t execute:
“`
> false && anoFunc(); < false
“`
These techniques can be useful in your web applications. You may encounter situations where you need to write several expressions based on which another function should run. Using shortcut logical operators (&& or ||), you can easily handle complex tasks.”
This translation captures the essence of using logical operators in JavaScript for conditional execution of functions based on expressions.
Post Comment