Array in javascript
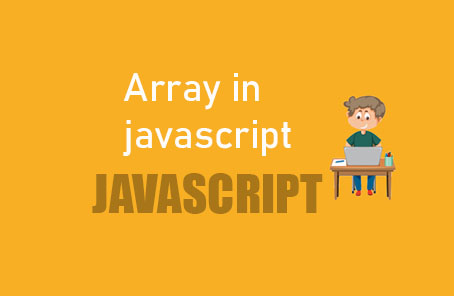
You want to store a lot of similar data. What can you do? You can store each separately with a variable for each.
Var name1 = “zonayed ahmed” ;
Var name2 = “jarif ahmed” ;
Var name3 = “david ahmed” ;
Var name4 = “masod ahmed” ;
Now, see, I want to store a lot of variables here, and it’s becoming cumbersome to store each variable one by one. Again, all the data here is somewhat similar, like names of several people. It doesn’t seem very efficient to do it this way. Maybe it’s okay to store variables separately for two or one variables. But when it becomes a lot, there’s a better solution than this. That’s using arrays. I’m going to discuss arrays here.
“Suppose there are many people with names like this:
Names = Rahim, Karim, Salam, Rafiq, Sharif ;
Now I want the name of Karim ;
Name[the second one] ;
Working this way, arrays, it’s somewhat helpful. Though it’ll be clearer with an actual code example. However, it’s necessary to understand the real concept. Array is a non-primitive data type. Array is a type of object. And what does object mean? Yes, object means it has some properties, which you can use to access a lot of things from the array. You’ll be able to learn about arrays. You’ll be able to modify arrays.
Declare an array in javascript:
The array can be declared in two ways. The first one is not used very often, but it’s good to know.
Var arrayName = new Array (“Rahim” , “Karim” , “Rafiq” , “jabbar” )
console.log(arrayName)
> (4) [“Rahim” , “Karim” , “Rafiq” , “jabbar”] ;
Look, here comes the output of all the data you’ve provided within you. Now, declaring like this isn’t very common. It’s also a bit difficult. You can make it easier and still use it most of the time.
Var arrayName = [“Rahim” , “Karim” , “Rafiq” , “Jabbar” ] ;
console.log(arrayName)
> (4) [“Rahim” , “Karim” , “Rafiq” , “Jabbar” ] ;
This is beyond the declaration phase. Let’s delve into something deeper. Earlier, I mentioned that the Chrome developer console can help you a lot in your JavaScript career. Now, if you look at the output you’ve got in the console, if you click on the triangle button on the left side, it will expand the array. Inside the array, you can see a lot of things to explore.
> arrayName
< (4) [“Rahim” , “Karim” , “Rafiq” , “Jabbar” ] ;
0: “Rahim” ,
1: “Karim” ,
2: “Rafiq” ,
3: “Jabbar”
Length: 4
>
Here, each piece of data is accompanied by a number. Yes, this is called an index number. Index numbers help you retrieve specific data from an array. Now why does counting start from 0? Well, in the world of programming, counting typically starts from 0. And array indices also start from 0. Now suppose you want to access Jabbar’s name. If it’s in the 4th position in normal counting, then if you start counting from 0, its position would be 3. And this 3 is its index number in the array. So, this is how you access a specific value from an array.
arrayName[3]
“Jabbar”
Now, if you want to change any specific value, you can also do it using these index numbers.
arrayName[3] = “Joneyed” ;
> (4) [“Rahim” , “Karim” , “Rafiq” , “Joneyed” ] ;
Ah, now we’re moving on from accessing items in an array to wanting to know more about the array itself. What do you want to know? Ah, you want to know how many items are there in the array. Look, in the same place, there’s a property called length, which tells you how many items are there in this array.
> length: 4
Here, properties inside ordinary objects are usually accessed using dot notation. This is called dot notation. If we want to access the number of items in an array now, we would write it like this
> arrayName.length = 4
So, what it’s showing here is that there are actually 4 items. Now, I talked about dot notation. There’s another one, which is bracket notation. Dot notation and bracket notation are used to access properties of all objects. So, it’s very important to know how to use them. If you want to access properties using bracket notation.
arrayName[ “length”] = 4
There is quite a similarity with the name, so I hope there won’t be a problem remembering dot notation and bracket notation. However, in the realm of JavaScript, dot notation is used more often.
Now, how can all items of an array be accessed? I think we discussed loops earlier. If we need to do the same task repeatedly, don’t we need to use loops? Yes, you can use a loop to access all items of an array.
Var arrayName = [“Rahim” , “Karim” , “Rafiq” , “Joneyed” ] ;
for( var i = 0; i < arrayName.length ; i++ ) {
console.log(“name “ + arrayName[i] ) ;
}
> Name : Rahim
Name : karim
Name : Rafiq
Name : Joneyed
>
If this code seems confusing, please pay attention to understand it better. Here, a for loop is being used, and each line follows a certain expression rule. Finally, it prints everything as desired at the end.
To add a new item to the end of the array:
If you want to add more items to an array where some items already exist, you can add new items either at the beginning or at the end. If you want to add items at the end.
arrayName.push (“newone”)
To remove an item from the end of an array.
We can also remove an item from an array either from the beginning or from the end. If we remove from the end, we use the pop method.
arrayName.pop()
To remove an item from the beginning of an array.
arrayName.shift()
When removing the first item, the index numbers of everyone will change.
To add an item to the beginning of an array.
If we want to add an item at the beginning in the same way.
arrayName.unshift(“newone”)
To know the index number of a specific item.
If you want to know the index number of any item, you can do it from the console, but sometimes you may need to know it from the code, in that case, we can use it.
arrayName.indexof(“karim”)
> 1
Post Comment