Advance . Map in javascript es6
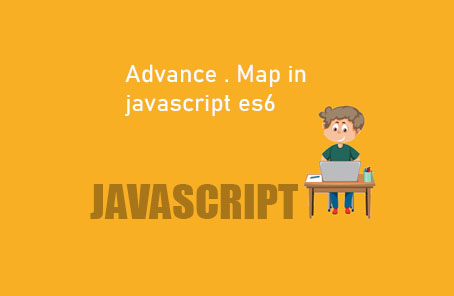
In JavaScript’s ES6, apart from some syntactic sugar, we haven’t seen anything significantly new so far. But now we are going to discuss something entirely new. Yes, this feature has been added completely new in ES6. It is called Map.
Don’t confuse this Map with the map method of arrays. The map method is an array method, but the Map we will discuss today is a data structure. This new data structure has been introduced to developers with the ES6 version of JavaScript. However, it is somewhat different from the object-type data structure. It has its own methods and characteristics. The Map can hold any type of data such as functions, booleans, integers, characters, and strings.
#### Creating a Map
Creating a new Map: If we want to create a new Map data structure, we can do it like this:
“`javascript
const zawad = new Map();
“`
That’s it! We have created a map named `zawad`. Now, a map contains keys and values. If we want to add new values to this map:
“`javascript
zawad.set(‘fullName’, ‘Zawad Ahmed’);
“`
To access any data from the map, we have another method. For example, to get the `fullName` from `zawad`:
“`javascript
zawad.get(‘fullName’); // “Zawad Ahmed”
“`
We can also check if a specific value exists in our map:
“`javascript
zawad.has(‘fullName’); // true
“`
Certainly! Here is the translation of the provided text into English with corrected JavaScript code:
—
Since our map has a key named `fullName`, it will return true:
“`javascript
zawad.has(‘fullName’); // true
“`
Now, if there is no such key, it will return false:
“`javascript
zawad.has(‘something’); // false
“`
We can also delete such a key-value pair from the map. First, let’s add a new key-value pair:
“`javascript
zawad.set(‘toBeRemoved’, ‘willBeRemoved’);
“`
Now, if we want to check if the new key-value pair has been added to the map:
“`javascript
zawad.has(‘toBeRemoved’); // true
“`
Now, we want to delete this `toBeRemoved` key:
“`javascript
zawad.delete(‘toBeRemoved’);
“`
Done! It has been deleted. Now if we check whether `toBeRemoved` exists:
“`javascript
zawad.has(‘toBeRemoved’); // false
“`
Because we just deleted it from our map.
—
Here is the translated version of the given text:
—
Now, if we want, we can clear the entire `zawad` map:
“`javascript
zawad.clear();
“`
Now if we check, we won’t find anything inside `zawad`:
“`javascript
console.log(zawad);
// Output: Map(0) {}
“`
Assume we have a map with a few elements like below:
“`javascript
const favorite = new Map();
“`
Now we will put some elements inside it:
“`javascript
favorite.set(‘name’, ‘Zonayed Ahmed’);
favorite.set(‘job’, ‘student’);
favorite.set(‘color’, ‘blue’);
favorite.set(‘os’, ‘ubuntu’);
favorite.set(‘mobileos’, ‘android’);
“`
Now, you will get all the data inside the `favorite` map:
“`javascript
console.log(favorite);
// Output: Map(5) {“name” => “Zonayed Ahmed”, “job” => “student”, “color” => “blue”, “os” => “ubuntu”, “mobileos” => “android”}
“`
If we want, we can also find out how many elements are inside the map using the `size` property of the map:
“`javascript
Favorite.size;
“`
Sure! Here’s the translated text:
—
Now we can even loop through a map:
“`javascript
favorite.forEach((value, key) => console.log(`Key is: ${key} and the value is: ${value}`));
“`
In the `forEach` method, we usually get the index number as the second argument, but in the case of a map, we get the map’s key:
“`javascript
favorite.forEach((value, key) => console.log(`Key is: ${key} and the value is: ${value}`));
// Output:
// Key is: name and the value is: Zonayed Ahmed
// Key is: job and the value is: student
// Key is: color and the value is: blue
// Key is: os and the value is: ubuntu
// Key is: mobileos and the value is: android
“`
Similarly, we can also loop using `for…of`:
“`javascript
for (let [key, value] of favorite.entries()) {
console.log(`Key is: ${key} and the value is: ${value}`);
}
// Output:
// Key is: name and the value is: Zonayed Ahmed
// Key is: job and the value is: student
// Key is: color and the value is: blue
// Key is: os and the value is: ubuntu
// Key is: mobileos and the value is: android
“`
With the map data structure:
1. We can easily loop through it.
2. We can get the size of the map.
3. Lastly, adding or deleting data from a map is very easy.
However, remember that since this is quite new, support for this data structure is limited. So, be sure to check if it is supported before using it anywhere.
Post Comment