What is IFE in javascript ?
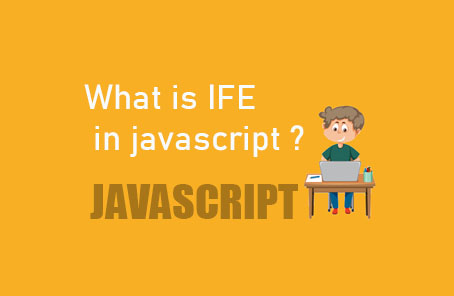
(Immediately Invoked Function Expressions)**
Usually, after creating a function, we need to call it to use it. But if we want to call the function immediately after creating it, we can use the IIFE (Immediately Invoked Function Expressions) technique.
In JavaScript, we can create a function in several ways:
“`javascript
function aDemoFunc() {
console.log(‘Hello World!’);
}
“`
or,
“`javascript
var aDemoFunc = function() {
console.log(‘Hello World!’);
}
“`
However, no matter how we create the function, we must call it to use it:
“`javascript
aDemoFunc();
“`
This will print “Hello World!” in the console.
But if we want to create and call the function immediately, we can use IIFE. Typically, in IIFE, we place the entire function inside the first brackets and call it with another set of brackets at the end. It will be clearer with an example. Suppose we want to create and call the above function immediately:
“`javascript
(function aDemoFunc() {
console.log(‘Hello World!’);
})();
“`
Here, the entire function goes inside the first brackets, and at the end, there are two more brackets where you can pass any arguments if the function has any. You will see that executing this prints “Hello World!” in the console immediately without needing to call the function separately.
Similarly, this works with function expressions as well. However, in this case, the return value of the function will be stored in that variable immediately:
“`javascript
var sum = (function() {
return 10 + 20;
})();
“`
If you check the value of `sum` after executing this, you will see it shows 30. You don’t need to call the function separately.
So, we’ve discussed IIFE, but how does it actually help us? Yes, let’s talk about that now.
Since the entire function is inside the first brackets, it is considered an expression rather than a statement. This allows you to create private functions. You cannot use this function outside or use anything inside it. So, if you want to maintain privacy or prevent the internal data of a function from being exposed, this can be very useful. For example:
“`javascript
(function aDemoFunc() {
console.log(‘Hello World!’);
})();
“`
This will print “Hello World!” in the console immediately, but if you try to call `aDemoFunc()` later, it will not work:
“`javascript
aDemoFunc()
Uncaught ReferenceError: aDemoFunc is not defined at <anonymous>:1:1
“`
The main purpose of a function is to solve a specific problem. When we create large projects, we try to make the entire project as modular as possible. We create different functions for different tasks. Suppose you have a function for score calculation in a JavaScript game, another for game control, and another for user interface changes. Using different functions for different tasks will make the project structure clean, and it will be easier to find bugs or for future developers to understand the coding pattern. This is called the modular pattern.
Another common problem we face is using many variables, and at some point, we can’t find a perfect name. Maybe this name has been used elsewhere in the program, or using a different name could cause confusion later. In such cases, creating private functions allows you to use variables with the same name in separate functions since they are completely private. This helps you write more semantic code.
In modular programming, if you want to get some value from a private function, you can use a function expression and return the value as an object or array from that function. You can use it later outside the function. For example:
“`javascript
var controller = (function() {
var a = {
name: ‘Zonayed Ahmed’,
uid: 1062
};
return a;
})();
“`
Here, an object is stored in `controller`, which is returned from the private function. This way, you can return anything after completing your task and use it later outside.
“`javascript
var interface = (function() {
return ‘Hello ‘ + controller.name;
})();
“`
Now, if you call `interface`, you will see it prints “Hello Zonayed Ahmed”:
“`javascript
> interface
< “Hello Zonayed Ahmed”
“`
So, this way, you can modularize your entire project. You can pass data from one function to another.
—
Post Comment