What is Logical Operators OR (||) in javascript ?
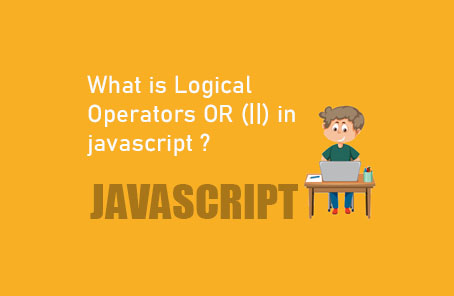
We learned about logical operators in the basics of JavaScript. Here, we will discuss two logical operators: OR (||) and AND (&&). Although they seem simple, they can perform much more complex tasks. Let’s delve into their usage.
Before we start with these, let’s discuss another aspect of JavaScript. In JavaScript, except for some special values, everything else (expressions) is considered a true expression. What does this mean? Let’s see the example below:
“`javascript
if (true) {
console.log(‘Print it!’);
}
“`
Now, this will print because our condition inside is true. If we set it to false, it won’t print:
“`javascript
if (false) {
console.log(‘Print it!’);
}
“`
Similarly, we usually write some expressions that return true or false instead of directly writing true or false. Decisions are made based on these expressions. Now, in the following type of expression, we might easily understand if it’s true or false:
“`javascript
if (10 > 5) {
console.log(‘Print it!’);
}
“`
But even if we write an expression like this:
“`javascript
if (‘anything’) {
console.log(‘Print it!’);
}
“`
If you run this, you will see your text printed:
“`javascript
if (‘anything’) {
console.log(‘Print it!’);
}
“`
Output:
“`
Print it!
“`
But what is the reason? Yes, the reason is that in JavaScript, most expressions are true, or we can say that any non-zero expression is true. Anything will do except the following:
- false (Boolean false, itself false)
- null
- NaN
- 0
- Empty (“”, ” or “`)
- undefined
- !anything True (an expression can be made false by preceding it with !)
If you use any of these for a condition, they will be considered false. Now, the logical operators || (OR) and && (AND) are mainly used with Boolean values. But due to some of their characteristics, many tasks can be shortcut using them. So let’s first explain what these two operators do in Bangla.
// OR operator If || or OR is between two expressions:
Go to class || Go for a walk
In Bangla, it translates to:
Go to class or go for a walk
Now, if you choose to go to class, you can’t go for a walk. That means, here you have only one option: either you go to class or go for a walk. Now, if you don’t go to class, then you can go for a walk. That means you can take the next option.
Using this technique, you can execute any one of two expressions. The concept is that if the first expression is true, the second one will not run. If the first one is false, the second one will run. Then the result will depend on whether the second one is true or false
“`javascript
console.log(true || false);
// > console.log(true || false);
// true
console.log(false || true);
// > console.log(false || true);
// true
console.log(false || false);
// > console.log(false || false);
// false
console.log(true || true);
// > console.log(true || true);
// true
“`
What will happen with just true or false? Yes, let’s now use this power to do something practical:
“`javascript
console.log(‘Go to Class’ || ‘Go to Visit’);
// > console.log(‘Go to Class’ || ‘Go to Visit’);
// Go to Class
“`
Here, since it found ‘Go to Class’ first, it printed that. But why? Yes, ‘Go to Class’ here also means true. And so, ‘Go to Class’ is printed here. It will become even clearer with this example:
“`javascript
console.log(undefined || ‘Go to Visit’);
// > console.log(undefined || ‘Go to Visit’);
// Go to Visit
“`
See, since the first value is false, it printed the second one. This is actually the real power of the OR operator.
—
Now how will this thing be useful in our real-life projects? Yes, our application may have some variables or values that might exist sometimes and might not exist at other times. Now we can use this operator to handle multiple variables or values. If one is not available, it will take another one. For example, in the case of variables:
“`javascript
const empty = ;
const something = ‘Eat’;
const work = empty || something;
“`
Now if we print `work` here:
“`javascript
console.log(work);
“`
See what is printed:
“`javascript
Eat
“`
Since the first variable `empty` has no value or is false, it moves to the next one. And since the next one has a value, it assigns that. Although we did this for only two variables here, you can do it for as many variables as you want. For example:
“`javascript
const aa = “”;
let bb; // cause const doesn’t allow empty variable initialization
const cc = ‘Hello’;
const dd = ‘A Lot’;
“`
If you print this, you will see the result:
“`javascript
console.log(aa || bb || cc || dd);
console.log(aa || bb || cc || dd);
“`
“`javascript
Hello
“`
Although I showed with two in most examples, you can use as many as you want like this. Let me show another example:
“`javascript
const user = {
name: ‘Zonayed Ahmed’
};
“`
—
Now you took another variable, in which you want to store this user’s name:
const userOrName = useror.name;
This is valid, and you can do this. Now you want to store the user’s age in another variable:
const userOrAgeTry = useror.age;
Even if you store it this way, you won’t get any errors. But if you want to store the age in this variable only if the user’s age is provided, and if not, then the variable should have a message that the user’s age is not found. We can do it like this:
let userOrAgeTry2;
if (useror.age) {
userOrAgeTry2 = useror.age;
} else {
userOrAgeTry2 = ‘User Age Not Found’;
}
Or, you might know that if there is only one line of code inside the if-else block, we can avoid the brackets. So our code will be like this:
if (useror.age) userOrAgeTry2 = useror.age;
else userOrAgeTry2 = ‘User Age Not Found’;
Now if you print this userOrAgeTry2 variable:
console.log(userOrAgeTry2);
> console.log(userOrAgeTry2);
User Age Not Found
Now this simple task can be done in one line using the OR operator:
const userOrAge = useror.age || ‘Age Not Found’;
“Busy! Job done. Now when we print this variable:
“`javascript
console.log(userOrAge); // console.log(userOrAge); Age Not Found
“`
Maybe a few lines have been omitted here, but I’ve kept the example simple. However, when you work on any web application, even such small techniques can be useful.
Again, if we want to run a function depending on the truth or falsehood of an expression, we can do it like this. Suppose we have a function:
“`javascript
const aFunc = () => {
console.log(‘Print Me!’);
}
“`
Now let’s run it based on another expression. If that expression is true, it won’t run:
“`javascript
true || aFunc(); // true
“`
But if that expression is false, we want this function to run:
“`javascript
false || aFunc(); // Print Me!
“`
This way, using this operator, you can do powerful tasks in moments. Just remember, even though I’ve shown you with two, you can take several together from your left, where you’ll find truth, until the operation continues.”
—
Post Comment