Error Handling in javascript
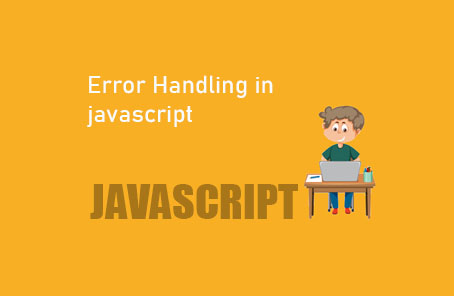
Errors are our everyday companions. If you write code, there will be errors. However, many of us think that errors are just errors, and we have no control over them. Yes, this is partially true but not entirely. Additionally, a good application should ensure that users are unaware of any errors. Users will not open the developer console like us to see if there is an error, so we need to incorporate the errors into a good user experience. This is why error handling is necessary. We will discuss this here.
In our application, there might be some code or functions that can result in errors. Therefore, we need to handle such code carefully. For this reason, JavaScript has some statements to handle such situations.
First, let’s look at a simple code without any error handling to see what output it gives:
“`javascript
console.aula (‘This is an error’);
“`
This will give a strict error:
“`javascript
> console.aula (‘This is an error’);
Uncaught TypeError: console.aula is not a function
at <anonymous>:1:9
“`
Now, this error might not have much effect on the code. But in our real web application, an error might cause the entire application to stop working properly, it might crash. Therefore, we need to handle such errors so that the web application can be prepared in advance for such errors. Then the application can function correctly. If an error occurs, it will be handled, and it won’t crash. If the error part is handled, the rest of the code or parts will function properly. We can handle errors using the following methods:
1. Try Statement: Enclose the part where there is a possibility of an error within this try block. If the code runs correctly, then it runs. But if there is an error, it will throw an error by itself:
“`javascript
try {
console.aula (‘This is an error’);
}
“`
Now, this try block code alone will not run; you will also need another statement with it.
——
**2. Catch Statement:** The job of the catch statement is to catch the error that is thrown. If there is no error, then the code inside this block will not execute. Basically, if there is an error, it needs to be handled here. In the previous try example, the try statement cannot work alone, it needs this catch statement:
“`javascript
try {
console.aula(‘This is an error’);
} catch (err) {
console.log(‘Do anything here’);
console.log(err);
}
“`
Now you will see how beautifully the output comes without showing a direct error:
“`
Do anything here
TypeError: console.aula is not a function
at <anonymous>:2:12
“`
The catch block is not for printing such errors; rather, you can write something nice here or give a message to the user that something else needs to be done. In short, you can handle the error here and give the user a good experience.
**3. Throw Statement:** With this statement, we can throw a custom error. Based on a condition in our code, we can show a custom error:
“`javascript
const age = 17;
try {
if (age < 18) {
throw ‘You are too young’;
} else {
console.log(‘You are adult’);
}
} catch (err) {
console.log(err);
}
“`
Output:
“`
You are too young
“`
finally statement: The code inside this statement will always run, whether there is an error or not:
try {
throw ‘An error’;
} catch (err) {
console.log(err);
} finally {
console.log(‘I will run always’);
}
// Output:
// An error
// I will run always
try {
console.log(‘No error’);
} catch (err) {
console.log(err);
} finally {
console.log(‘I will run always’);
}
// Output:
// No error
// I will run always
This finally is usually used to take some mandatory action after trying a code block. Whether the code runs successfully or throws an error, the error is caught in the catch block, but after finishing all my work, I must perform a certain task. And in such situations, this finally is useful.
**Error Object:**
In JavaScript, there is also a built-in Error object. And an object means it has properties. We can create such an Error object using the `Error()` constructor method. Earlier, we used `throw` to throw a custom text to show an error. But to give the error a more realistic form, we can create a more elegant Error object using the `Error()` constructor:
“`javascript
const anError = new Error(‘This is an error object’);
“`
Now, if you run and see our newly created `anError` Error object:
“`javascript
console.log(anError);
“`
It appears as a real error:
“`javascript
> console.log(anError);
Error: This is an error object
at <anonymous>:1:17
“`
Where can we use this? Yes, in the above `throw` example, we threw a custom error, which was just a piece of text. But now, using this Error constructor method, we can throw an error more elegantly like a real error:
“`javascript
const ageNew = 17;
try {
if (ageNew < 18) {
throw new Error(‘You are too young’);
} else {
console.log(‘You are adult’);
}
} catch (err) {
console.log(err);
}
“`
This time, it throws the error like a real error:
“`javascript
> const ageNew = 17;
try {
if (ageNew < 18) {
throw new Error(‘You are too young’);
} else {
console.log(‘You are adult’);
}
} catch (err) {
console.log(err);
}
Error: You are too young
at <anonymous>:4:13
“`
Post Comment